Object-Oriented Programming in Love2D(Lua)
Posted: Sun Nov 28, 2021 5:12 am
I got interested in Love2D and Lua and decided to give it a try.
So in order to get familiar both with Lua and Love2D, I code out an easy sample:
Project Struture:
demo
|-ball.lua
|-main.lua
ball.lua
```
Ball = {
x = 0,
y = 0,
xSpeed = 0,
ySpeed = 0,
ballRadius = 0,
r = 0,
g = 0,
b = 0
}
function Ball:new(x, y, xSpeed, ySpeed, ballRadius, r, g, b)
t = {
x = x,
y = y,
xSpeed = xSpeed,
ySpeed = ySpeed,
ballRadius = ballRadius,
r = r,
g = g,
b = b
}
setmetatable(t, self)
self.__index = self
return t
end
function Ball:move()
self.x = self.x + self.xSpeed
self.y = self.y + self.ySpeed
end
function Ball:changeColor()
self.r = love.math.random(0, 255)
self.g = love.math.random(0, 255)
self.b = love.math.random(0, 255)
print('color: ' .. self.r .. ' ' .. self.g .. ' ' .. self.b)
end
function Ball:checkEdges()
if self.x + self.ballRadius > love.graphics.getWidth() or self.x - self.ballRadius < 0 then
self.xSpeed = self.xSpeed * -1
Ball:changeColor()
end
if self.y + self.ballRadius> love.graphics.getHeight() or self.y - self.ballRadius < 0 then
self.ySpeed = self.ySpeed * -1
Ball:changeColor()
end
end
function Ball:show()
love.graphics.setColor(self.r, self.g, self.b)
love.graphics.ellipse('fill', self.x, self.y, self.ballRadius)
end
```
main.lua
```
require "ball"
local ball = nil
local x, y
function love.load()
x = love.graphics.getWidth() / 2
y = love.graphics.getHeight() / 2
ball = Ball:new(x, y, 2, 3.5, 20, 255, 255, 255)
end
function love.update(dt)
Ball.move(ball)
Ball.checkEdges(ball)
end
function love.keypressed(key)
if key == 'escape' then
love.event.quit()
end
end
function love.draw()
love.graphics.setBackgroundColor(0, 0, 0)
Ball.show(ball)
end
```
so basically it's just a ball bouncing around when it hits the edges.
Everything seems fine except for the function Ball:changeColor()
I want the ball to change color everytime it hits the edges, but this isn't working. is it something wrong with the function changeColor() ?
here's a snapshot of the demo:
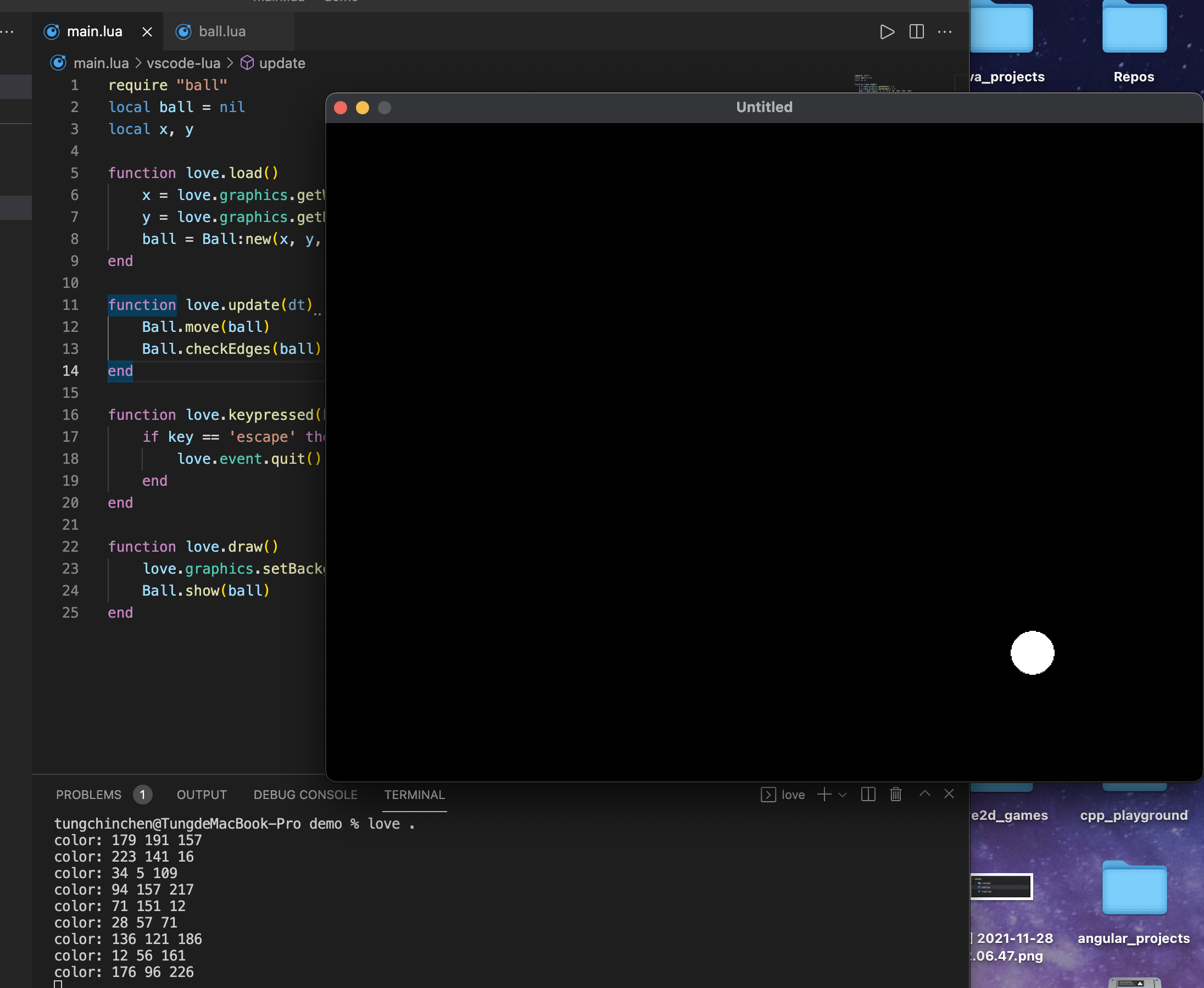
the function did trigger and the rgb color values did change, but the ball itself didn't change color, any help is appreciated!
So in order to get familiar both with Lua and Love2D, I code out an easy sample:
Project Struture:
demo
|-ball.lua
|-main.lua
ball.lua
```
Ball = {
x = 0,
y = 0,
xSpeed = 0,
ySpeed = 0,
ballRadius = 0,
r = 0,
g = 0,
b = 0
}
function Ball:new(x, y, xSpeed, ySpeed, ballRadius, r, g, b)
t = {
x = x,
y = y,
xSpeed = xSpeed,
ySpeed = ySpeed,
ballRadius = ballRadius,
r = r,
g = g,
b = b
}
setmetatable(t, self)
self.__index = self
return t
end
function Ball:move()
self.x = self.x + self.xSpeed
self.y = self.y + self.ySpeed
end
function Ball:changeColor()
self.r = love.math.random(0, 255)
self.g = love.math.random(0, 255)
self.b = love.math.random(0, 255)
print('color: ' .. self.r .. ' ' .. self.g .. ' ' .. self.b)
end
function Ball:checkEdges()
if self.x + self.ballRadius > love.graphics.getWidth() or self.x - self.ballRadius < 0 then
self.xSpeed = self.xSpeed * -1
Ball:changeColor()
end
if self.y + self.ballRadius> love.graphics.getHeight() or self.y - self.ballRadius < 0 then
self.ySpeed = self.ySpeed * -1
Ball:changeColor()
end
end
function Ball:show()
love.graphics.setColor(self.r, self.g, self.b)
love.graphics.ellipse('fill', self.x, self.y, self.ballRadius)
end
```
main.lua
```
require "ball"
local ball = nil
local x, y
function love.load()
x = love.graphics.getWidth() / 2
y = love.graphics.getHeight() / 2
ball = Ball:new(x, y, 2, 3.5, 20, 255, 255, 255)
end
function love.update(dt)
Ball.move(ball)
Ball.checkEdges(ball)
end
function love.keypressed(key)
if key == 'escape' then
love.event.quit()
end
end
function love.draw()
love.graphics.setBackgroundColor(0, 0, 0)
Ball.show(ball)
end
```
so basically it's just a ball bouncing around when it hits the edges.
Everything seems fine except for the function Ball:changeColor()
I want the ball to change color everytime it hits the edges, but this isn't working. is it something wrong with the function changeColor() ?
here's a snapshot of the demo:
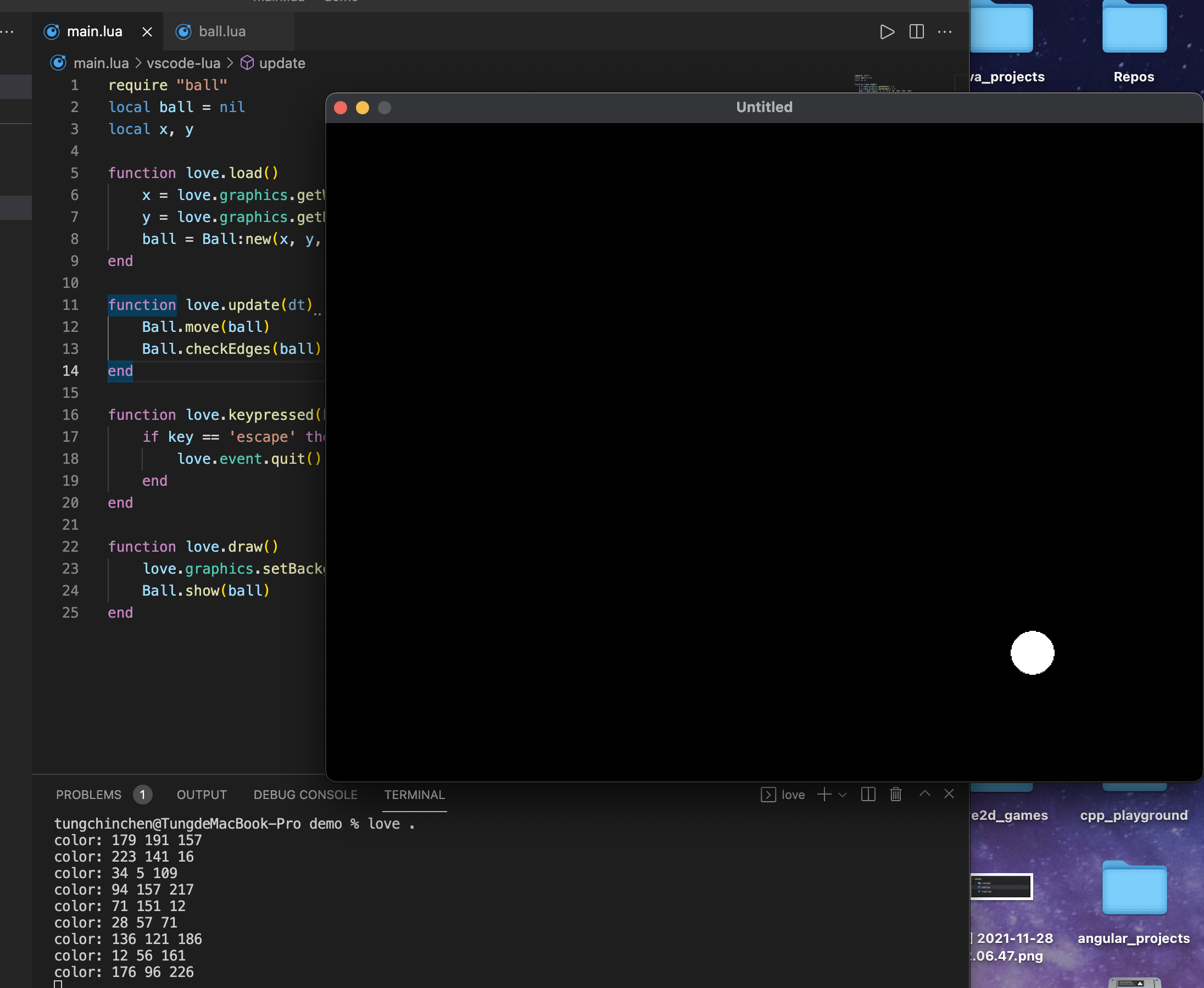
the function did trigger and the rgb color values did change, but the ball itself didn't change color, any help is appreciated!