Assigning an image to consecutive tables using 'for loop'
Posted: Tue Aug 27, 2019 1:29 pm
Hello, I'm new to programming. Currently, I'm in need of help with my code.
How I want the application to work: Create 5 images of column to fill up the window horizontally.
How I wrote the code to accomplish my idea:
-First, create table 'coltable'. Next, make table 'i'.
-The image file of the column is 32x128 in dimensions. The window size is 320x256. By using ' love.graphics.scale( 2,2 ) ', the column would be 64x256. So, to fill the window with columns, a number of exactly 5 columns would be needed. Moreover, they have to be 64px apart (from top left corner). Additionally, I wanted the 5 columns to be 5 individual tables with each of them having their own image file.
-I didn't want to manually type in the coordinates for each column so I tried using 'for loop'. I create value 'colx' and assign it a 0 ( colx = 0 ). After each loop, 64 is added to colx ( colx = colx + 64 ).
-With each loop, a column will be drawn next to the preceding one, eventually, the screen would be filled with columns.
-The loop goes from 1 to 5. At the end of the loop, I should have these indexes: coltable[1].img , coltable[2].img, coltable[3].img, coltable[4].img , coltable[5].img . 5 columns holding their own images.
The code:
The result: Instead of 5 columns, there is only a single image moving horizontally, which means that the 'for loop' works.
What it should look like:
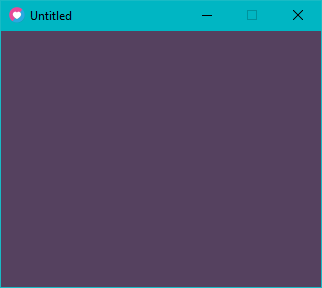
What it looked like:
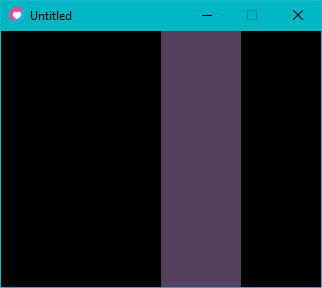
(The photo is static but the column does move horizontally in motion)
I'm not sure what I understood wrong. Thanks for reading.
How I want the application to work: Create 5 images of column to fill up the window horizontally.
How I wrote the code to accomplish my idea:
-First, create table 'coltable'. Next, make table 'i'.
-The image file of the column is 32x128 in dimensions. The window size is 320x256. By using ' love.graphics.scale( 2,2 ) ', the column would be 64x256. So, to fill the window with columns, a number of exactly 5 columns would be needed. Moreover, they have to be 64px apart (from top left corner). Additionally, I wanted the 5 columns to be 5 individual tables with each of them having their own image file.
-I didn't want to manually type in the coordinates for each column so I tried using 'for loop'. I create value 'colx' and assign it a 0 ( colx = 0 ). After each loop, 64 is added to colx ( colx = colx + 64 ).
-With each loop, a column will be drawn next to the preceding one, eventually, the screen would be filled with columns.
-The loop goes from 1 to 5. At the end of the loop, I should have these indexes: coltable[1].img , coltable[2].img, coltable[3].img, coltable[4].img , coltable[5].img . 5 columns holding their own images.
The code:
Code: Select all
--Create table to store individual columns
coltable = {}
--Values to insert individual columns to coltable
i = {}
--Coordinate to draw columns
colx = 0
function love.load()
--Change window size
--Change filter
love.window.setMode( 320, 256 )
love.graphics.setDefaultFilter( 'nearest', 'nearest' )
end
--Create individual columns
--Insert image to individual columns
for i = 1, 5 do
coltable[i] = {}
coltable[i].img = love.graphics.newImage('col.png')
end
function love.draw()
--Scale 200%
love.graphics.scale( 2, 2 )
--Draw column sprites
for i = 1, 5 do
love.graphics.draw(coltable[i].img, colx, 0)
colx = colx + 2
-- Supposedly 64 but I add only 2 so it's easier to see how it fails to work properly in action
end
end
What it should look like:
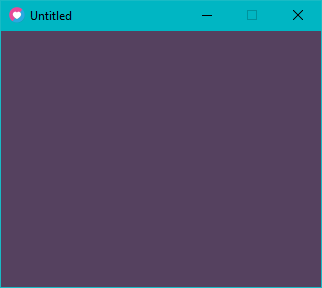
What it looked like:
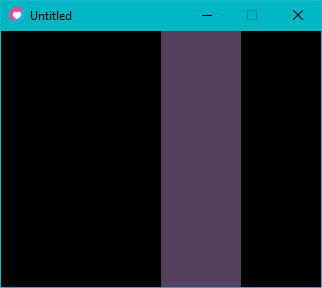
(The photo is static but the column does move horizontally in motion)
I'm not sure what I understood wrong. Thanks for reading.