TicTacToe Question
Posted: Thu Jan 10, 2019 1:09 am
Hello there fellow lua-ers... love-ers.
I recently got into lua and was wondering the best way about making the tictactoe board for tictactoe... Except mine is called kittytactoe but that's besides the point. So far, I have done this.
TLDR I made a rectangle, filled it, and made some lines but it was a very.. how do I say, random process due to the pixel approximations for where the lines should go that I ended up making. I'm pretty sure this isn't the best of ways to go about making the board itself.
This is a mess, I know. It's pretty bad. The menu for this is broken but I decided to move on to the actual
game mechanics and go back to that later.
I also have a question about mousepressed. The way I am using it here makes the circle appear on the screen but of course it's just in random pixelated areas that can be anywhere which is why I was wondering if I needed to fix my game board or fix my mousepressed. I'll have a screenshot so you can vizualize what i'm talking about.
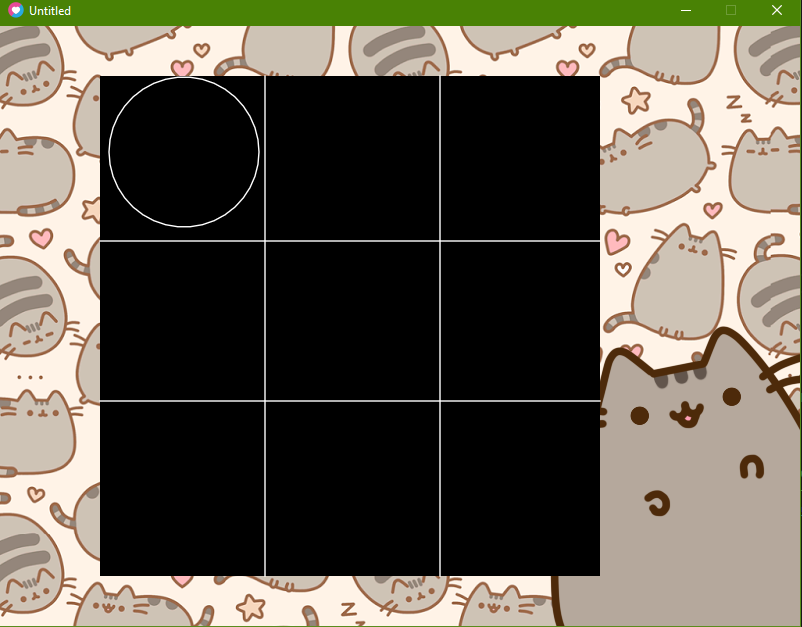
I recently got into lua and was wondering the best way about making the tictactoe board for tictactoe... Except mine is called kittytactoe but that's besides the point. So far, I have done this.
Code: Select all
local MENU_PLAY = 0
local MENU_SETTINGS = 1
local MENU_QUIT = 2
local MENU_MAXIMUM = 2
local MENU_MINIMUM = 0
local menu_selection = 0
local change_menu = false
local main_menu = false
local play_game = false
local back = false
local quit = false
local mouseClicked = false
-- SETTINGS
local settings_selection = 0
local SETTINGS_MAXIMUM = 0
local SETTINGS_MINIMUM = 0
local SETTINGS_VOLUME = 0
function love.load()
kitty = love.graphics.newImage("kitty.jpg")
cursor = love.graphics.newImage("paw.png")
game = love.graphics.newImage("gameBackground.png")
pusheen = love.graphics.newImage("sticker.png")
pizzaPiece = love.graphics.newImage("pizza.png")
donutPiece = love.graphics.newImage("donuts.png")
font = love.graphics.newFont("Babylove.ttf", 50)
xPush = 550
yPush = 300
printx = 0
printy = 0
end
function love.draw()
love.window.setFullscreen(true, "desktop")
mainMenu()
if (menu_selection == MENU_QUIT) then
quitGame()
elseif (menu_selection == MENU_SETTINGS) then
settings()
elseif (menu_selection == MENU_PLAY) then
gameBoard()
gamePieces()
end
end
function mainMenu()
-- DRAW MAIN MENU SCREEN
love.graphics.setColor(1, 1, 1, 1)
love.graphics.draw(kitty)
love.graphics.setFont(font)
love.graphics.setColor(0, 0, 0, 1)
love.graphics.print("Play", 100, 150)
love.graphics.print("Settings", 100, 200)
love.graphics.print("Quit", 100, 250)
love.graphics.setColor(1, 1, 1, 1)
if (menu_selection == MENU_PLAY) then
love.graphics.draw(cursor, 10, 120, 0, 0.1, 0.1)
elseif (menu_selection == MENU_SETTINGS) then
love.graphics.draw(cursor, 10, 170, 0, 0.1, 0.1)
elseif (menu_selection == MENU_QUIT) then
love.graphics.draw(cursor, 10, 220, 0, 0.1, 0.1)
end
end
function settings()
-- DRAW THE SETTINGS SCREEN
if (change_menu == true) then
main_menu = false
love.graphics.setColor(1, 1, 1, 1)
love.graphics.draw(kitty)
love.graphics.setColor(0, 0, 0, 1)
love.graphics.print("Volume", 100, 150)
love.graphics.setColor(1, 1, 1, 1)
if (settings_selection == SETTINGS_VOLUME) then
love.graphics.draw(cursor, 10, 120, 0, 0.1, 0.1)
end
end
end
function quitGame()
-- QUIT IF QUIT IS SELECTED
if (menu_selection == MENU_QUIT) then
quit = true
end
end
function previousWindow()
if (back == true) then
main_menu = true
love.graphics.setColor(1, 1, 1, 1)
love.graphics.draw(kitty)
love.graphics.setFont(font)
love.graphics.setColor(0, 0, 0, 1)
love.graphics.print("Play", 100, 150)
love.graphics.print("Settings", 100, 200)
love.graphics.print("Quit", 100, 250)
love.graphics.setColor(1, 1, 1, 1)
if (menu_selection == MENU_PLAY) then
love.graphics.draw(cursor, 10, 120, 0, 0.1, 0.1)
elseif (menu_selection == MENU_SETTINGS) then
love.graphics.draw(cursor, 10, 170, 0, 0.1, 0.1)
elseif (menu_selection == MENU_QUIT) then
love.graphics.draw(cursor, 10, 220, 0, 0.1, 0.1)
end
end
end
function gameBoard()
-- DRAW THE GAMEBOARD SCREEN
if (play_game == true) then
main_menu = false
love.graphics.setColor(1, 1, 1, 1)
love.graphics.draw(game)
love.graphics.draw(pusheen, xPush, yPush)
love.graphics.setFont(font)
love.graphics.setColor(0, 0, 0, 1)
love.graphics.rectangle("fill", 100, 50, 500, 500)
love.graphics.setColor(1, 1, 1, 1)
-- VERTICAL LINES
love.graphics.line(265, 50, 265, 550)
love.graphics.line(440, 50, 440, 550)
-- HORIZONTAL LINES
love.graphics.line(100, 215, 600, 215)
love.graphics.line(100, 375, 600, 375)
end
end
function gamePieces()
if (play_game == true) then
main_menu = false
if (mouseClicked == true) then
-- love.graphics.draw(donutPiece, 120, 75, 0, 0.30, 0.30)
-- love.graphics.draw(pizzaPiece, printx, printy, 0, 0.85, 0.85)
love.graphics.circle("line", printx, printy, 75)
end
end
end
function love.keypressed(key)
if key == "escape" then
if (main_menu == true) then
love.event.quit()
else
back = true
end
elseif key == "up" then
menu_selection = math.max(menu_selection - 1, MENU_MINIMUM)
elseif key == "down" then
menu_selection = math.min(menu_selection + 1, MENU_MAXIMUM)
elseif key == "return" then
if (menu_selection == MENU_SETTINGS) then
print("Enter key pressed")
change_menu = true
elseif (menu_selection == MENU_PLAY) then
play_game = true
elseif (menu_selection == MENU_QUIT) then
love.event.quit()
end
end
end
function love.mousepressed(x, y, button)
if button == 1 then
mouseClicked = true
printx = x
printy = y
end
end
This is a mess, I know. It's pretty bad. The menu for this is broken but I decided to move on to the actual
game mechanics and go back to that later.
I also have a question about mousepressed. The way I am using it here makes the circle appear on the screen but of course it's just in random pixelated areas that can be anywhere which is why I was wondering if I needed to fix my game board or fix my mousepressed. I'll have a screenshot so you can vizualize what i'm talking about.
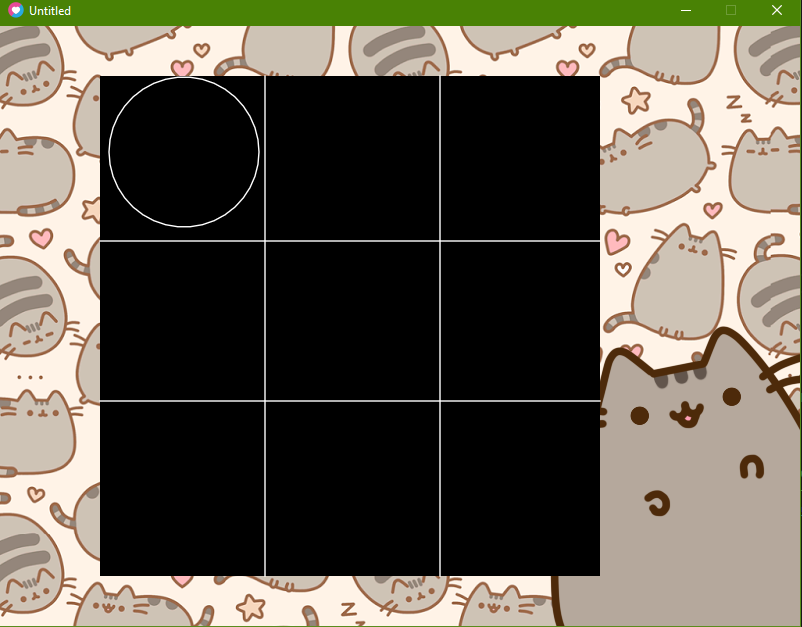