I create the body like this, which is using https://github.com/SSYGEN/windfield. Essentially the collider is "a composition of a single body, fixture and shape".
Code: Select all
function factory.ship(gameState, x, y, size, callback)
local world = gameState.physics.world
-- create a triangle
local shipBody = world:newPolygonCollider(
{-size,-(2*size/3), 0,size, size,-(2*size/3)}
)
shipBody:setPosition(x, y)
local weaponWidth = size*0.2
-- place each weapon on each side of the ship
shipWeapon1 = world:newRectangleCollider(
x - size/2 - weaponWidth/2,
-size/3,
weaponWidth,
size*1.2)
shipWeapon2 = world:newRectangleCollider(
x + size/2 - weaponWidth/2,
-size/3,
weaponWidth,
size*1.2)
-- place joint in centre of player, the weapons and the main
-- body should collide with each other
world:addJoint('WeldJoint', shipWeapon1, shipBody, x, y, true)
world:addJoint('WeldJoint', shipWeapon2, shipBody, x, y, true)
-- make collisions inellastic
shipBody:setRestitution(0.2)
shipWeapon1:setRestitution(0.2)
shipWeapon2:setRestitution(0.2)
-- minimise unwanted spinning, especially on welded weapons
shipBody:setAngularDamping(20)
shipWeapon1:setAngularDamping(20)
shipWeapon2:setAngularDamping(20)
-- make movement slow down
shipBody:setLinearDamping(0.1)
shipWeapon1:setLinearDamping(0.1)
shipWeapon2:setLinearDamping(0.1)
...
Code: Select all
if gameState.inputs.up.down then
local angle = player:getAngle() + math.pi/2
local dx, dy = 50*math.cos(angle), 50*math.sin(angle)
player:applyLinearImpulse(dx, dy)
end
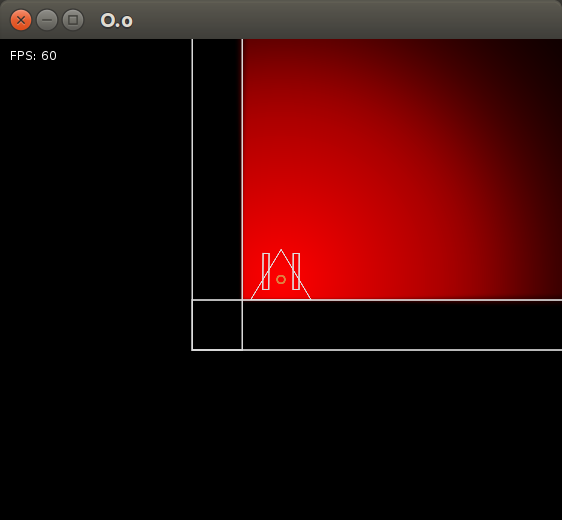