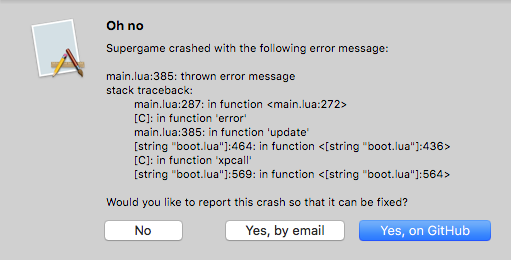
Clicking the email button opens a new email with email-address, error message, stacktrace, version and edition filled in:
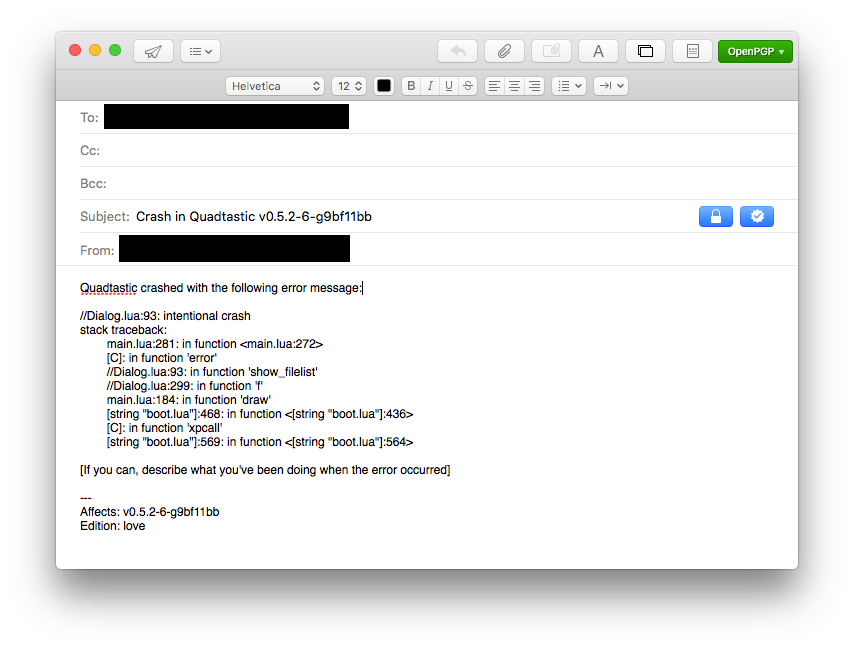
Clicking the GitHub button opens this page, again with all crash details filled in:
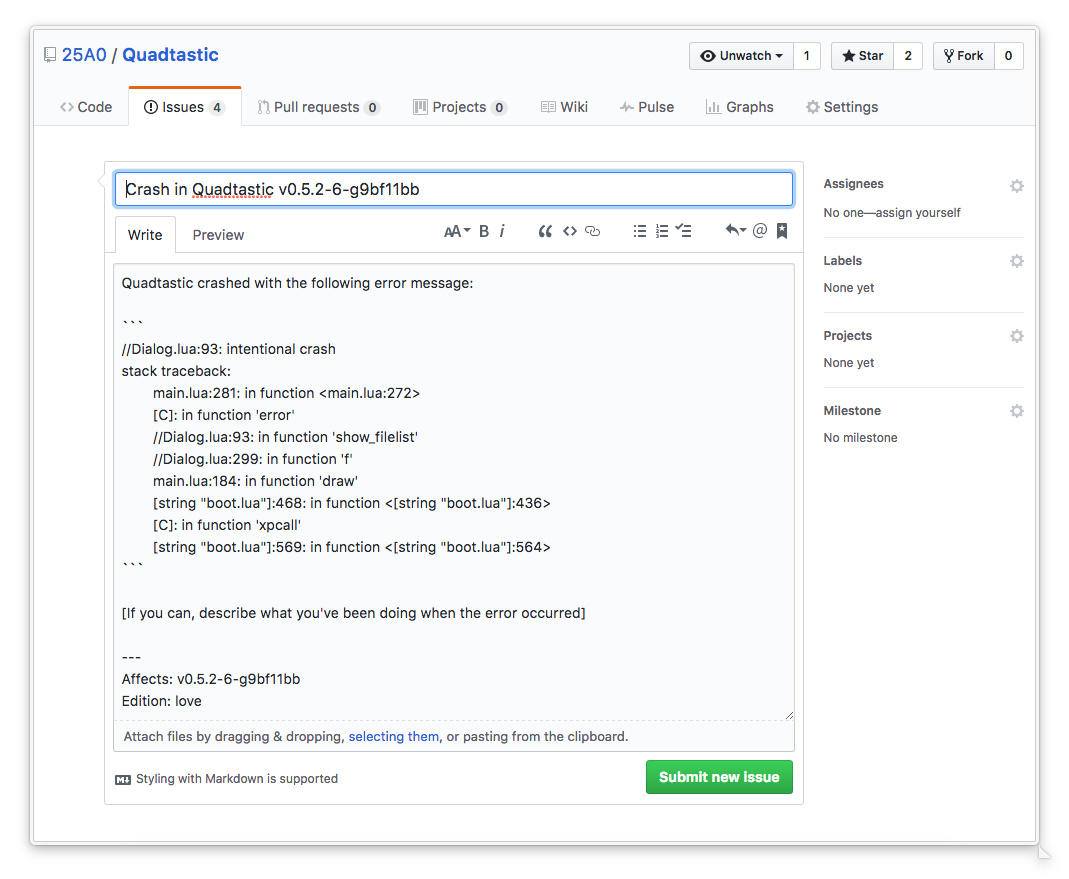
If you want to use it in your project, all you need to do is fill in the details at the top of the function, and put it in your main.lua. Hopefully it will be obvious how to remove the github option if you don't need it, or replace it with the issue tracker of your choice.
I hope it's not inappropriate to post this here; it's by far not a full tool or library, but I wanted to share it to hear what you think about the approach, and I'm curious what other people do to collect crash reports.
A neat thing about this approach is that it gives you a way to get in touch with the user who reported the crash, either via the email-address they used or via their GitHub account. This is handy if you need more information to reproduce the crash, or simply if you want to thank them for reporting the crash.
I used this for my tool Quadtastic and received my first crash report today with a bug that I might have missed otherwise.
Code: Select all
function love.errhand(error_message)
local app_name = "Supergame"
local version = "game version"
local github_url = "https://www.github.com/user/repo" -- no trailing slash
local email = "your-email@provider.tld"
local edition = love.system.getOS()
local dialog_message = [[
%s crashed with the following error message:
%s
Would you like to report this crash so that it can be fixed?]]
local titles = {"Oh no", "Oh boy", "Bad news"}
local title = titles[love.math.random(#titles)]
local full_error = debug.traceback(error_message or "")
local message = string.format(dialog_message, app_name, full_error)
local buttons = {"Yes, on GitHub", "Yes, by email", "No"}
local pressedbutton = love.window.showMessageBox(title, message, buttons)
local function url_encode(text)
-- This is not complete. Depending on your issue text, you might need to
-- expand it!
text = string.gsub(text, "\n", "%%0A")
text = string.gsub(text, " ", "%%20")
text = string.gsub(text, "#", "%%23")
return text
end
local issuebody = [[
%s crashed with the following error message:
%s
[If you can, describe what you've been doing when the error occurred]
---
Affects: %s
Edition: %s]]
if pressedbutton == 1 then
-- Surround traceback in ``` to get a Markdown code block
full_error = table.concat({"```",full_error,"```"}, "\n")
issuebody = string.format(issuebody, app_name, full_error, version, edition)
issuebody = url_encode(issuebody)
local subject = string.format("Crash in %s %s", app_name, version)
local url = string.format("%s/issues/new?title=%s&body=%s",
github_url, subject, issuebody)
love.system.openURL(url)
elseif pressedbutton == 2 then
issuebody = string.format(issuebody, app_name, full_error, version, edition)
issuebody = url_encode(issuebody)
local subject = string.format("Crash in %s %s", app_name, version)
local url = string.format("mailto:%s?subject=%s&body=%s",
email, subject, issuebody)
love.system.openURL(url)
end
end

*edit 2* Forgot to mention that email and GitHub issues will already contain all necessary information