I have seen people use math.floor but I do not quite understand it.
I want the program to round to the nearest integer and return it.
I don't mind if someone just gives me a rounding system as long as I understand it
How do I round a number in Lua?
Forum rules
Before you make a thread asking for help, read this.
Before you make a thread asking for help, read this.
Re: How do I round a number in Lua?
'math.floor' always rounds a number down. To round to the nearest integer, you can do the following:
You can also make a wrapper function for this:
Hope this helps. 
Code: Select all
roundedInteger = math.floor(float + 0.5)
Code: Select all
function round(n)
return math.floor(n + 0.5)
end
roundedInteger = round(float)

- zorg
- Party member
- Posts: 3470
- Joined: Thu Dec 13, 2012 2:55 pm
- Location: Absurdistan, Hungary
- Contact:
Re: How do I round a number in Lua?
Comprehensive post inbound; 
math.floor always rounds all numbers to the next whole number towards negative infinity;
math.ceil always rounds all numbers to the next whole number towards positive infinity;
There are two more options for "basic" rounding, towards zero, and away from zero; the former is usually called truncation, the latter doesn't really have a name, but both can be implemented quite easily.
The bigger problems come from when a number is exactly .5 away from any integer, since its nearest integers are the same in both directions; with the above four, it's a non-issue, since they always round towards the directions i mentioned, regardless of the fractional part, that's why they're also called directed rounding functions; for the latter one though, you need to choose a tie-breaking function.
What tjakka linked above is the one that "rounds up" (towards positive infinity), and is the most common one (well, either this or the towards both infinities, but the difference only comes up with the negative numbers).
Here's the two additional functions that aren't built-in to lua, for reference. (copied from this previous post i made regarding this.)These were the fastest implementations for the two functions back when i wrote the previous post, should still be the fastest ones even now.
Also, here's the relevant wikipedia link, and to be honest, this image is awesome in showing what i mean (it's an interactive svg, click the image to go there):
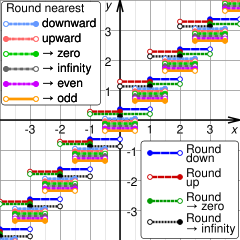
The four on the right are the four i mentioned first, directed rounding; the six on the left are various tie-breaking rules for rounding to the "nearest integer". One isn't limited to those six tie-breakers either, the wikipedia article lists at least two more. (stochastic, i.e. random, and alternating)

math.floor always rounds all numbers to the next whole number towards negative infinity;
math.ceil always rounds all numbers to the next whole number towards positive infinity;
There are two more options for "basic" rounding, towards zero, and away from zero; the former is usually called truncation, the latter doesn't really have a name, but both can be implemented quite easily.
The bigger problems come from when a number is exactly .5 away from any integer, since its nearest integers are the same in both directions; with the above four, it's a non-issue, since they always round towards the directions i mentioned, regardless of the fractional part, that's why they're also called directed rounding functions; for the latter one though, you need to choose a tie-breaking function.
What tjakka linked above is the one that "rounds up" (towards positive infinity), and is the most common one (well, either this or the towards both infinities, but the difference only comes up with the negative numbers).
Here's the two additional functions that aren't built-in to lua, for reference. (copied from this previous post i made regarding this.)
Code: Select all
math.trunc = function(n) return n >= 0.0 and n-n% 1 or n-n%-1 end -- rounds towards zero, away from both infinities.
math.round = function(n) return n >= 0.0 and n-n%-1 or n-n% 1 end -- rounds away from zero, towards both infinities.
Also, here's the relevant wikipedia link, and to be honest, this image is awesome in showing what i mean (it's an interactive svg, click the image to go there):
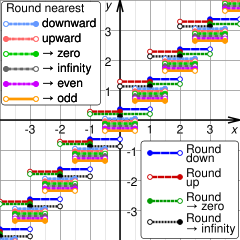
The four on the right are the four i mentioned first, directed rounding; the six on the left are various tie-breaking rules for rounding to the "nearest integer". One isn't limited to those six tie-breakers either, the wikipedia article lists at least two more. (stochastic, i.e. random, and alternating)
Me and my stuff
True Neutral Aspirant. Why, yes, i do indeed enjoy sarcastically correcting others when they make the most blatant of spelling mistakes. No bullying or trolling the innocent tho.

Who is online
Users browsing this forum: Bing [Bot], slime and 7 guests