Joystick input correction code
Posted: Sat Mar 28, 2015 1:31 pm
The input from the sticks isn't as accurate as the uninitiated might think.
This program demonstrates and solves the inaccuracy.
The purple line originating from the middle shows the raw input data from the controller.
The darkened area in the middle is the deadzone that is associated with the raw input.
The lighter circle is the rectified input.
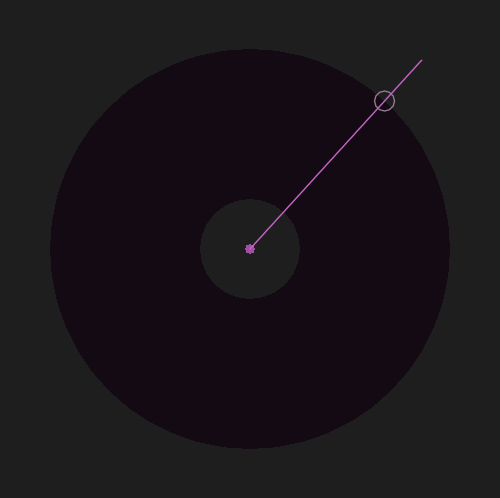
When you roll your stick around a full circle, you can see that it doesn't make a smooth circle; it makes this puffy pillowy shape -- which isn't how it should be, so it needs some code to rectify that.
This program demonstrates and solves the inaccuracy.
Code: Select all
function love.load()
deadzone = 0.25 -- adjustable, my pretty worn controller needs to have this as high as 0.3
love.graphics.setBackgroundColor(30, 30, 30)
joystick = love.joystick.getJoysticks()[1]
s = {}
end
function love.update()
s.ax, s.ay = joystick:getAxes() -- ax and ay are the actual raw values from the controller
local extent = math.sqrt(math.abs(s.ax * s.ax) + math.abs(s.ay * s.ay))
local angle = math.atan2(s.ay, s.ax)
if (extent < deadzone) then
s.x, s.y = 0, 0 -- x and y are the rectified inputs
else
extent = math.min(1, (extent - deadzone) / (1 - deadzone))
s.x, s.y = extent * math.cos(angle), extent * math.sin(angle)
end
end
function love.draw()
-- bullseye
love.graphics.setColor(20, 10, 20)
love.graphics.circle("fill", 250, 250, 200, 48)
love.graphics.setColor(30, 30, 30)
love.graphics.circle("fill", 250, 250, 200 * deadzone, 24)
-- actual values indicator line
love.graphics.setColor(160, 80, 160)
love.graphics.circle("fill", 250, 250, 5, 8)
love.graphics.setColor(200, 100, 200)
love.graphics.line(250, 250, 250 + (s.ax * 200), 250 + (s.ay * 200))
-- fixed location indicator circle
love.graphics.setColor(150, 120, 150)
love.graphics.circle("line", 250 + (s.x * 200), 250 + (s.y * 200), 10, 12)
end
The darkened area in the middle is the deadzone that is associated with the raw input.
The lighter circle is the rectified input.
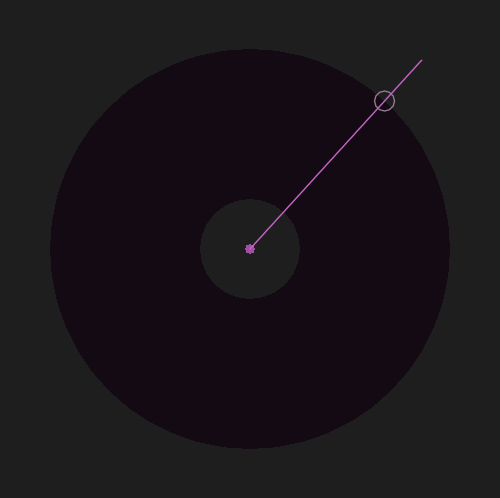
When you roll your stick around a full circle, you can see that it doesn't make a smooth circle; it makes this puffy pillowy shape -- which isn't how it should be, so it needs some code to rectify that.