I've been using Tiled Map Editor to define a static background image for the level, and then placing polygons for the boundaries in that level. I then wrote a small class that takes Tiled's exported lua file, and creates the proper polygon shapes in HardonCollider.
This all works fine. The issue is when my player actually collides with said polygons, things start to freak out. I originally thought it was because of my collision resolution code (which is admittedly extremely basic), but the curious thing is that the behavior only happens some of the time. In fact, simply closing and re-opening the game is enough to change which polygons act like this.
Here's an example of the wonkyness:
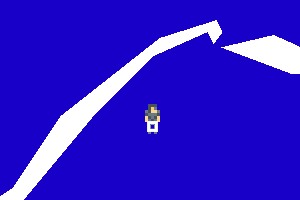
And here is the same set of polygons working after a restart:
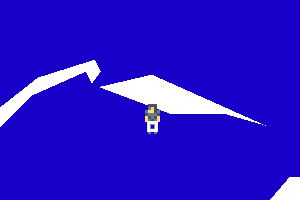
My collision resolution code:
Code: Select all
function on_collide(dt, shape_one, shape_two, dx, dy)
-- print("collide")
local playerCollide = false
if shape_one == player.physics then
playerCollide = true
elseif shape_two == player.physics then
playerCollide = true
end
if playerCollide and player.moving then
player.physics:move(0, dy)
player.physics:move(dx, 0)
local x, y = player.physics:center()
player.x = x - (player.width / 2)
player.y = y - player.height + (player.width / 2)
end
end
Code: Select all
if object.shape == "polyline" then
local line = {}
local linePoints = {}
for k, segment in pairs(object.polyline) do
table.insert(linePoints, object.x + segment.x)
table.insert(linePoints, object.y + segment.y)
end
line.physics = physics:addPolygon(unpack(linePoints))
physics:addToGroup("boundries", line.physics)
table.insert(self.boundries, line)
end