Code Doodles!
Posted: Wed Apr 02, 2014 1:14 pm
Code Doodles (as I call them), are short codes for small applications. The whole code is in a main.lua, and isn't very big. I don't want to set any rules to it, but I try to create them in an hour or 2. I started making them when helping a friend with programming, and me playing around with the small examples. Now I am making them every lunch break. I got 4 right now. Here they are! Maybe they inspire you to doodle some code yourself? Then share as you like!
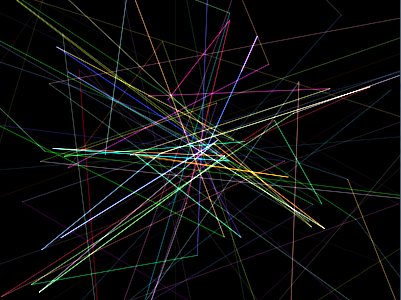
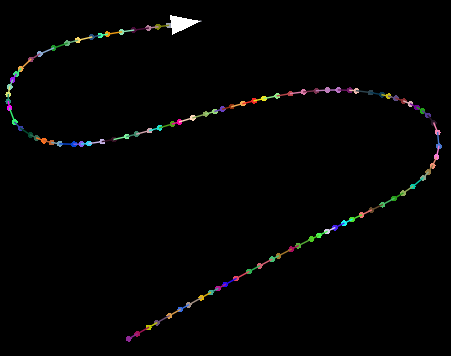
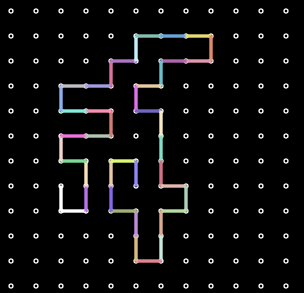
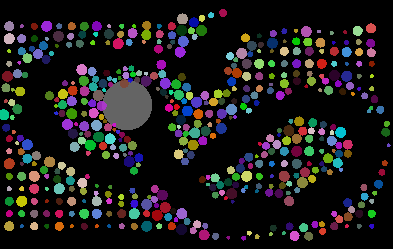
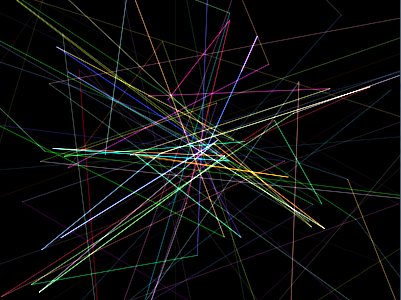
Code: Select all
function love.load()
math.randomseed(os.time(),love.mouse.getX(),love.mouse.getY())
xposition = love.mouse.getX()
yposition = love.mouse.getY()
a = {x = 200, y = 200 }
b = {x = xposition, y = yposition}
angle = math.atan2(b.y-a.y,b.x-a.x)
speed = 0
balls = {}
colors = {{255,255,255}}
directions = {}
currentcolor = {255,255,255}
tocenter = false
rotate = 0
rotatespeed = 0
end
function love.update(dt)
if not love.mouse.isDown("l") then
rotate = rotate + rotatespeed * dt
angle = math.atan2(b.y-a.y,b.x-a.x)
xposition = love.mouse.getX()
yposition = love.mouse.getY()
a.x = a.x + math.cos(angle) * getDistance(a.x,a.y,b.x,b.y) * 50 * dt
a.y = a.y + math.sin(angle) * getDistance(a.x,a.y,b.x,b.y) * 50 * dt
end
if getDistance(a.x,a.y,b.x,b.y) < 200 then
table.insert(balls,{x=b.x,y=b.y})
table.insert(colors,{unpack(currentcolor)})
table.insert(directions,angle)
if tocenter then
b.x, b.y = 400,300
else
b.x, b.y = math.random(800),math.random(600)
end
tocenter = not tocenter
angle = math.atan2(b.y-a.y,b.x-a.x)
currentcolor = {math.random(255),math.random(255),math.random(255)}
end
for i,v in ipairs(balls) do
v.x = v.x + math.cos(directions[i]) * 300 * dt
v.y = v.y + math.sin(directions[i]) * 300 * dt
end
if #balls>100 then
table.remove(balls,1)
table.remove(colors,1)
table.remove(directions,1)
end
end
function love.draw()
-- love.graphics.setColor(currentcolor)
-- love.graphics.circle("fill",a.x,a.y,10,10)
-- love.graphics.setColor(255,255,255)
-- love.graphics.circle("line",b.x,b.y,10,10)
-- for i,v in ipairs(balls) do
-- love.graphics.setColor(unpack(colors[i]))
-- love.graphics.circle("fill", v.x, v.y, 10, 20)
-- end
love.graphics.translate(400,300)
love.graphics.rotate(rotate)
love.graphics.translate(-400,-300)
love.graphics.setLineWidth(2)
love.graphics.setBlendMode("additive")
for i=1,#balls do
--love.graphics.setLineWidth((i*5)/#balls)
local r,g,b = unpack(colors[math.ceil(i)])
love.graphics.setColor(r,g,b,i*255/#balls)
if i<#balls then
love.graphics.line(balls[i].x,balls[i].y,balls[i+1].x or a.x,balls[i+1].y or a.y)
else
love.graphics.line(balls[i].x,balls[i].y,a.x, a.y)
end
end
end
function love.mousepressed(x, y, button)
if button == "l" then
b.x, b.y = x,y
angle = math.atan2(b.y-a.y,b.x-a.x)
speed = 100
end
if button == "wu" then
rotatespeed = rotatespeed + 0.1
elseif button == "wd" then
rotatespeed = rotatespeed - 0.1
end
end
function getDistance(x1,y1,x2,y2)
local d = x1 - x2
local e = y1 - y2
d = d^2
e = e^2
f = d + e
f = math.sqrt(f)
return f
end
function love.keypressed(k)
if k == " " then
love.window.setFullscreen(not love.window.getFullscreen())
end
end
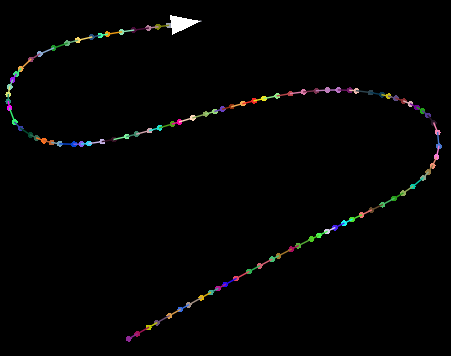
Code: Select all
function love.load()
math.randomseed(os.time(),love.mouse.getX(),love.mouse.getY())
head = {x=400,y=300}
tail = {}
colors = {}
directions = {}
for i=1,100 do
table.insert(tail,{x=-6000+math.random(12000),y=-6000+math.random(12000)})
table.insert(colors,{math.random(255),math.random(255),math.random(255)})
table.insert(directions,math.random(math.pi*2))
end
angle = 0
timer = 0.2
end
function love.update(dt)
if love.keyboard.isDown(" ") then
for i,v in ipairs(tail) do
v.x = v.x + math.cos(directions[i]) * 500 * dt
v.y = v.y + math.sin(directions[i]) * 500 * dt
end
else
for i,v in ipairs(tail) do
if i == 1 then
if getDistance(head,v) > 30 then
local ang = math.atan2(head.y-v.y,head.x-v.x)
v.x = v.x + math.cos(ang) * 500 * dt
v.y = v.y + math.sin(ang) * 500 * dt
end
else
local j = i-1
if getDistance(tail[j],v) > 15 then
local ang = math.atan2(tail[j].y-v.y,tail[j].x-v.x)
v.x = v.x + math.cos(ang) * 500 * dt
v.y = v.y + math.sin(ang) * 500 * dt
end
end
end
end
if love.keyboard.isDown("left") then
angle = angle - 8 * dt
end
if love.keyboard.isDown("right") then
angle = angle + 8 * dt
end
if love.keyboard.isDown("up") then
head.x = head.x + 500 * math.cos(angle) * dt
head.y = head.y + 500 * math.sin(angle) * dt
end
if head.x > 800 then
head.x = 0
end
if head.y > 600 then
head.y = 0
end
if head.x < 0 then
head.x = 800
end
if head.y < 0 then
head.y = 600
end
timer = timer - dt
if timer < 0 then
local temp = colors[1]
table.remove(colors,1)
table.insert(colors,temp)
timer = 0.05
end
end
function love.draw()
for i,v in ipairs(tail) do
love.graphics.setColor(colors[i][1],colors[i][2],colors[i][3])
if i == 1 then
love.graphics.line(v.x,v.y,head.x,head.y)
else
love.graphics.line(v.x,v.y,tail[i-1].x,tail[i-1].y)
end
love.graphics.circle("fill", v.x, v.y, 3, 10)
end
love.graphics.setColor(255,255,255)
love.graphics.push()
love.graphics.translate(head.x, head.y)
love.graphics.rotate(angle)
love.graphics.polygon("fill", 0, 0, -30, -10, -30,10)
love.graphics.pop()
end
function getDistance(a,b)
return math.sqrt((a.x-b.x)^2+(a.y-b.y)^2)
end
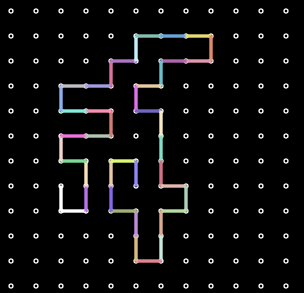
Code: Select all
function love.load()
math.randomseed(os.time()+love.mouse.getX()+love.mouse.getY())
lines = {{x=400,y=300},{x=400,y=325}}
timer = 0.5
colors = {{255,255,255},{255,255,255}}
end
function love.update(dt)
timer = timer - dt
if timer < 0 then
newLine()
timer = 0.01
end
end
function love.draw()
love.graphics.setLineWidth(1)
love.graphics.setColor(255,255,255)
for i=1,713 do
i = i-1
love.graphics.circle("line", 25+(i%31)*25, 25+(math.floor(i/31))*25, 2, 10)
end
love.graphics.setLineWidth(3)
for i,v in ipairs(lines) do
if i~=#lines then
love.graphics.setColor(unpack(colors[i]))
love.graphics.line(v.x,v.y,lines[i+1].x,lines[i+1].y)
end
end
end
function newLine()
local result = false
local warning = 0
local tries = {1,2,3,4}
while not result and warning < 5 do
warning = warning + 1
local direction = tries[math.random(#tries)]
if direction == 1 then
local newplace = lines[#lines].x + 25
local allowed = true
if newplace < 800 then
for i,v in ipairs(lines) do
if v.x == newplace and v.y == lines[#lines].y then
allowed = false
table.remove(tries,direction)
break
end
end
if allowed then
table.insert(lines,{x=newplace,y=lines[#lines].y})
table.insert(colors,{100+math.random(155),100+math.random(155),100+math.random(155)})
result = true
break
end
end
end
if direction == 2 then
local newplace = lines[#lines].y + 25
local allowed = true
if newplace < 600 then
for i,v in ipairs(lines) do
if v.x == lines[#lines].x and v.y == newplace then
allowed = false
table.remove(tries,direction)
break
end
end
if allowed then
table.insert(lines,{x=lines[#lines].x,y=newplace})
table.insert(colors,{100+math.random(155),100+math.random(155),100+math.random(155)})
result = true
break
end
end
end
if direction == 3 then
local newplace = lines[#lines].x - 25
local allowed = true
if newplace > 0 then
for i,v in ipairs(lines) do
if v.x == newplace and v.y == lines[#lines].y then
allowed = false
table.remove(tries,direction)
break
end
end
if allowed then
table.insert(lines,{x=newplace,y=lines[#lines].y})
table.insert(colors,{100+math.random(155),100+math.random(155),100+math.random(155)})
result = true
break
end
end
end
if direction == 4 then
local newplace = lines[#lines].y - 25
local allowed = true
if newplace > 0 then
for i,v in ipairs(lines) do
if v.x == lines[#lines].x and v.y == newplace then
allowed = false
table.remove(tries,direction)
break
end
end
if allowed then
table.insert(lines,{x=lines[#lines].x,y=newplace})
table.insert(colors,{100+math.random(155),100+math.random(155),100+math.random(155)})
result = true
break
end
end
end
end
end
function love.keypressed(key)
if key == " " or key == "r" then
love.load()
end
end
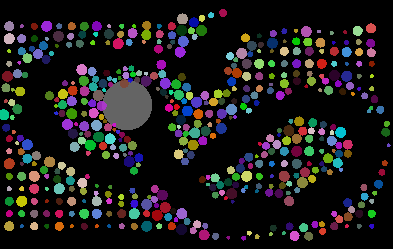
Code: Select all
function love.load()
math.randomseed(os.time()+love.mouse.getX()+love.mouse.getY())
balls = {{x=1000,y=1000,size=60,angle=0,force=100}}
colors = {{255,255,255,100}}
for i=1,510 do
i = i-1
table.insert(balls,{x=25+(i%30)*25,y=100+math.floor(i/30)*25,size=3+math.random(8),angle=0,force=0})
table.insert(colors,{math.random(255),math.random(255),math.random(255),220})
end
toggle = false
end
function love.update(dt)
if love.mouse.isDown("r") then
balls[1].size = 1000
else
balls[1].size = 50
end
balls[1].x = love.mouse.getX()
balls[1].y = love.mouse.getY()
if love.mouse.isDown("l") then
for i,v in ipairs(balls) do
if i~=1 then
local j = i-2
local p = {x=25+(j%30)*25,y=100+math.floor(j/30)*25}
local angle = math.atan2(p.y-v.y,p.x-v.x)
v.x = v.x + math.cos(angle) * 100 * dt
v.y = v.y + math.sin(angle) * 100 * dt
v.force = 0
end
end
else
if toggle then
for i,b1 in ipairs(balls) do
for j,b2 in ipairs(balls) do
if b1~=b2 and i~=1 then
if getDistance(b1,b2) < b1.size + b2.size then
local angle = math.atan2(b2.y-b1.y,b2.x-b1.x)
b1.x = b1.x - math.cos(angle) * (b1.size + b2.size)*100/getDistance(b1,b2) * dt
b1.y = b1.y - math.sin(angle) * (b1.size + b2.size)*100/getDistance(b1,b2) * dt
end
end
end
end
else
for i,b1 in ipairs(balls) do
if i~=1 then
for j,b2 in ipairs(balls) do
if b1~=b2 then
if getDistance(b1,b2) < b1.size + b2.size then
b1.angle = math.atan2(b2.y-b1.y,b2.x-b1.x)
b1.force = (b1.size + b2.size)*100/getDistance(b1,b2)
end
end
end
b1.x = b1.x - math.cos(b1.angle) * b1.force * dt
b1.y = b1.y - math.sin(b1.angle) * b1.force * dt
b1.force = math.max(0,b1.force - 100 * dt)
end
end
end
end
end
function love.draw()
for i,v in ipairs(balls) do
love.graphics.setColor(unpack(colors[i]))
love.graphics.circle("fill", v.x, v.y, v.size, 50)
end
end
function getDistance(a,b)
return math.sqrt((a.x-b.x)^2+(a.y-b.y)^2)
end
function love.keypressed(key)
if key == " " then
toggle = not toggle
end
end