rotate an image in the direction of the mouse
Forum rules
Before you make a thread asking for help, read this.
Before you make a thread asking for help, read this.
- luislasonbra
- Citizen
- Posts: 60
- Joined: Sun Jun 24, 2012 1:57 pm
rotate an image in the direction of the mouse
hi how I can make the red barrel follow the mouse or look
-
- Prole
- Posts: 7
- Joined: Mon Jan 07, 2013 8:48 pm
Re: rotate an image in the direction of the mouse
A little trigonometry should do the trick. You have a triangle like this, where B is the barrel and M is the position of your mouse cursor.
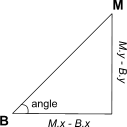
You know the coordinates of both B and M, so you can calculate two of the sides of the triangle: M.x - B.x and M.y - B.y. Now you can find out the angle you need using the arc tangent, or in Lua:
Note that I'm assuming a conventional coordinate system in my example. The computer screen uses a coordinate system where the y-axis is flipped, so you'll have to compensate for that, but the principle is the same.
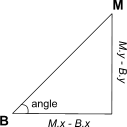
You know the coordinates of both B and M, so you can calculate two of the sides of the triangle: M.x - B.x and M.y - B.y. Now you can find out the angle you need using the arc tangent, or in Lua:
Code: Select all
math.atan2(M.y - B.y, M.x - B.x)
-
- Party member
- Posts: 235
- Joined: Sat Dec 15, 2012 6:54 am
Re: rotate an image in the direction of the mouse
Here is a heavily commented example:
Hopefully that helps!
EDIT:
Code: Select all
--set barrel image
barrelImage = love.graphics.newImage("barrel.png")
--set some basic variables for barrel
barrelDirection = 0
barrelX = 200
barelY = 200
--Remember: images rotate around their origin point
--Since the default origin point is (0,0), that means that the image
--would rotate around it's upper-left corner, meaning that it will
--move around in a big circle as it rotates.
--If you set the origin point to the center of the image (image's width/2, image's height/2)
--then the image won't appear to orbit around as it rotates.
--This also means that you'll have to adjust the x and y position you set accordingly
--e.g. if the barrel's x origin is 16 and it's x is 100, then the image will be actually
--be displayed at x position 84 (100 - 16 = 84). So take this into account when
--you set the barrel's position.
barrelOriginX = barrelImage:getWidth()/2
barrelOriginY = barrelImage:getHeight()/2
function love.update()
--use the handy "find rotation" function that I added to the bottom of this code
barrelDirection = findRotation(barrelX,barrelY,love.mouse.getX(),love.mouse.getY())
end
function love.draw()
--draw the barrel
--see https://love2d.org/wiki/love.graphics.draw for info on the love.graphics.draw()
--function's arguments
love.graphics.draw(barrelImage,barrelX,barrelY,barrelDirection,1,1,barrelOriginX,barrelOriginY)
end
--basic "find rotation" math function
function findRotation(x1,y1,x2,y2)
return math.atan2(y2 - y1, x2 - x1)
end
EDIT:
Yep, exactly!William Willing wrote:A little trigonometry should do the trick. You have a triangle like this, where B is the barrel and M is the position of your mouse cursor.
You know the coordinates of both B and M, so you can calculate two of the sides of the triangle: M.x - B.x and M.y - B.y. Now you can find out the angle you need using the arc tangent, or in Lua:
Note that I'm assuming a conventional coordinate system in my example. The computer screen uses a coordinate system where the y-axis is flipped, so you'll have to compensate for that, but the principle is the same.Code: Select all
math.atan2(M.y - B.y, M.x - B.x)
Re: rotate an image in the direction of the mouse
If you are drawing the barrel with löve's line function, you don't need angles. You can operate on the coordinate vectors directly.
Calculate the difference vector between mouse position and barrel position and divide this by it's length. Then multiply by the length of the barrel.
Calculate the difference vector between mouse position and barrel position and divide this by it's length. Then multiply by the length of the barrel.
Check out my blog on gamedev
- luislasonbra
- Citizen
- Posts: 60
- Joined: Sun Jun 24, 2012 1:57 pm
Re: rotate an image in the direction of the mouse
William Willing wrote:A little trigonometry should do the trick. You have a triangle like this, where B is the barrel and M is the position of your mouse cursor.
You know the coordinates of both B and M, so you can calculate two of the sides of the triangle: M.x - B.x and M.y - B.y. Now you can find out the angle you need using the arc tangent, or in Lua:
Note that I'm assuming a conventional coordinate system in my example. The computer screen uses a coordinate system where the y-axis is flipped, so you'll have to compensate for that, but the principle is the same.Code: Select all
math.atan2(M.y - B.y, M.x - B.x)
hello and have not been able to fix the problem
when I put the code
Code: Select all
Math.atan2 (m.y - B.y, m.x - B.x) [/ code]
the gun is not pointed at the sight
Re: rotate an image in the direction of the mouse
This line only calculates the angle between barrel and mouse. You have to store it in a variable and give it as a parameter in the graphics.draw function.luislasonbra wrote: hello and have not been able to fix the problem
when I put the code
the gun is not pointed at the sightCode: Select all
Math.atan2 (m.y - B.y, m.x - B.x)
Read the code in scutheotaku's post. There it is explained in detail.
Check out my blog on gamedev
- luislasonbra
- Citizen
- Posts: 60
- Joined: Sun Jun 24, 2012 1:57 pm
Re: rotate an image in the direction of the mouse
micha wrote:This line only calculates the angle between barrel and mouse. You have to store it in a variable and give it as a parameter in the graphics.draw function.luislasonbra wrote: hello and have not been able to fix the problem
when I put the code
the gun is not pointed at the sightCode: Select all
Math.atan2 (m.y - B.y, m.x - B.x)
Read the code in scutheotaku's post. There it is explained in detail.
Hi I have solved the problem thanks
Re: rotate an image in the direction of the mouse
Hello I have tried to use this code but I get an error.
this is my code and when I run it I get this error.
man.lua:12: attempted to perform arithmetic on global 'mY' (a nil value)
Code: Select all
function man.load()
man = love.graphics.newImage("sprite/Char.png")
manx = 0
many = 0
manxvel = 10
manyvel = 10
findRotation = math.atan2(mY - many, mX - manx)
mandirection = findRotation(manx,many,love.mouse.getX(),love.mouse.getY())
manOriginX = man:getWidth()/2
manOriginY = man:getHeight()/2
local mX, mY = love.mouse.getPosition()
end
man.lua:12: attempted to perform arithmetic on global 'mY' (a nil value)
- Jasoco
- Inner party member
- Posts: 3727
- Joined: Mon Jun 22, 2009 9:35 am
- Location: Pennsylvania, USA
- Contact:
Re: rotate an image in the direction of the mouse
First, you made the mX and mY variables local to the love.load function.
Second, grabbing the mouse coordinates in love.load is useless for the most part. It should be in love.update. And if you're going to want to access mX or mY at any point elsewhere, then they should not be local.
Second, grabbing the mouse coordinates in love.load is useless for the most part. It should be in love.update. And if you're going to want to access mX or mY at any point elsewhere, then they should not be local.
Re: rotate an image in the direction of the mouse
I tried this
But I got the same error
Code: Select all
function love.update(dt)
findRotation = math.atan2(mouseY - many, mX - manx)
man.update()
mouseY = love.mouse.getY()
end
Last edited by Heeloo on Wed Jun 25, 2014 2:12 am, edited 1 time in total.
Who is online
Users browsing this forum: Amazon [Bot], Google [Bot] and 6 guests