Code Doodles!
Re: Code Doodles!
Good stuff, everybody. Here is mine.
Use arrow keys to generate waves in x- or y-direction (need to hold down for one or two seconds, until nice waves emerge).- Attachments
-
doodle1.love
- (1.67 KiB) Downloaded 346 times
Check out my blog on gamedev
-
- Citizen
- Posts: 67
- Joined: Fri Mar 07, 2014 8:16 pm
Re: Code Doodles!
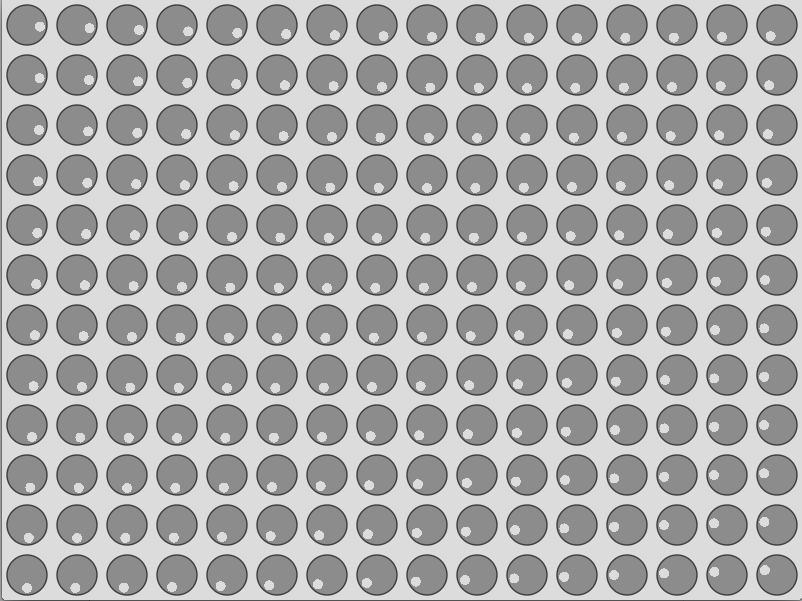
This doodle displays clocks going at different rates. If you watch closely, you can see the clocks all go into phase, and then break and go to seemingly random positions. If you rather a youtube to explain this than the code, here it is, it is the same visual concept:
http://youtu.be/V87VXA6gPuE
To change the visual style, hit any key.
Sorry this is so similar to the one before; I saw your screen shot and thought of this exhibit I read about awhile ago which had many clocks reacting to external input. Turns out, that is slightly similar to what you did. I guess we don't all need to do unique things.
Code: Select all
clocks = {}
uselines = true
function makeClock(x,y,rate)
local c = {}
c.x = x
c.y = y
c.rate = rate
c.rot = 0
function c:draw()
love.graphics.setColor(140,140,140)
love.graphics.circle( "fill", x, y, 20, 50 )
love.graphics.setColor(60,60,60)
love.graphics.circle( "line", x, y, 20, 50 )
local dx,dy = math.cos(math.rad(self.rot))*13, math.sin(math.rad(self.rot))*13
if uselines then
love.graphics.setColor(60,60,60)
love.graphics.line( x,y,x+dx,y+dy )
else
love.graphics.setColor(220,220,220)
love.graphics.circle( "fill", x+dx,y+dy, 5, 20 )
end
end
function c:update(td)
self.rot = self.rot+ self.rate*td
end
return c
end
function love.load()
for x=0,love.graphics.getWidth()-40,50 do
for y=0,love.graphics.getHeight()-40,50 do
local c = makeClock(x+25,y+25,50+x+y)
table.insert(clocks,c)
end
end
love.graphics.setBackgroundColor( 220, 220, 220 )
end
function love.keypressed(key,isrepeat)
uselines = not uselines
end
function love.update(td)
for _,c in ipairs(clocks) do
c:update(td)
end
end
function love.draw()
for _,c in ipairs(clocks) do
c:draw()
end
end
Re: Code Doodles!
Too bad we can't do 3D???
A 2 hour doodle.
(Don't know why it rotates faster the mode sides it has.)
A 2 hour doodle.
(Don't know why it rotates faster the mode sides it has.)
- Attachments
-
doodle3d.love
- Simple multisided cylinder
- (1.68 KiB) Downloaded 378 times
- Sheepolution
- Party member
- Posts: 264
- Joined: Mon Mar 04, 2013 9:31 am
- Location: The Netherlands
- Contact:
Re: Code Doodles!
That is so cool! 3D stuff in LÖVE is awesome.Ref wrote:Too bad we can't do 3D???
A 2 hour doodle.
(Don't know why it rotates faster the mode sides it has.)
Re: Code Doodles!
Really off topic but ...
Took Helvecta's image and wrapped it around a cylinder using xXxMonkEyMaNxXx's shader.
Code's really a hack job.
Just a quick look-see for what it would take.
Best
Took Helvecta's image and wrapped it around a cylinder using xXxMonkEyMaNxXx's shader.
Code's really a hack job.
Just a quick look-see for what it would take.
Best
- Attachments
-
frustration.love
- Simple 3D texturing
- (27.12 KiB) Downloaded 351 times
- HugoBDesigner
- Party member
- Posts: 403
- Joined: Mon Feb 24, 2014 6:54 pm
- Location: Above the Pocket Dimension
- Contact:
Re: Code Doodles!
So long (not much) without a doodle... I've got one I was trying to make for quite a long time (grid alignment maths made it take longer...). It is a doodle of falling blocks and... No, it's just this. But, as in my 2 previous doodles, you can make a lot of things via keyboard. Here are the "controls" of this doodle:
"R": Randomizes all the colors of all blocks.
Enter: Toggles economic mode (the outline, only. It doesn't makes much difference, though).
"P": Toggles "pyramid-looking" details (don't turn them off. They look really good
).
Down/"S"/Delete: Removes the last line of blocks.
Space: Spawns a block in a random place.
Left mouse click: Spawns a block in the same column of the click.
Right mouse click: Randomizes the color of the block you clicked on.
Mouse wheel: Increases/decreases the simulation speed.
Middle mouse click: Resets the simulation speed.
EDIT: Just like my last doodle, I made some improvements in this one. Now, by pressing "F", you can change the block's shapes (blocks, circles, hexagons or triangles). The new code:
And a new screenshot:
"R": Randomizes all the colors of all blocks.
Enter: Toggles economic mode (the outline, only. It doesn't makes much difference, though).
"P": Toggles "pyramid-looking" details (don't turn them off. They look really good

Down/"S"/Delete: Removes the last line of blocks.
Space: Spawns a block in a random place.
Left mouse click: Spawns a block in the same column of the click.
Right mouse click: Randomizes the color of the block you clicked on.
Mouse wheel: Increases/decreases the simulation speed.
Middle mouse click: Resets the simulation speed.
Code: Select all
-- LÖVE Code Doodle #3
-- by HugoBDesigner
function love.load()
-- GOOD VARIABLE S TO MESS AROUND WITH:
recsize = 40 --Size, in pixels, of the blocks
maxheight = 12 --The maximum height of a pile of blocks
fakeblockmaxtime = 2 --The time it takes to remove a line after it surpasses the maximum height
gridw = math.floor(800/recsize)
gridh = math.floor(600/recsize)
maxheight = gridh-maxheight
fakeblocks = {}
fakeblocktimer = 0
simspeed = 1
grid = {}
gridwait = {}
minspawntime = .05
maxspawntime = .25
minspeed = 3
maxspeed = 12
spawntime = math.random(minspawntime, maxspawntime)
for x = 1, gridw do
grid[x] = {}
gridwait[x] = false
for y = 1, gridh do
grid[x][y] = false
end
end
economic = false
pyramid = true
fallingblocks = {}
love.graphics.setBackgroundColor(255, 255, 255, 255)
end
function love.update(ndt)
local dt = ndt*simspeed
if fakeblocktimer > 0 then
fakeblocktimer = fakeblocktimer - dt
if fakeblocktimer <= 0 then
fakeblocktimer = 0
removeline()
end
return
end
for i, v in pairs(fallingblocks) do
v.y = v.y + v.speed*dt
if v.y >= v.dy and not v.die then
grid[v.x][v.dy] = v.color
gridwait[v.x] = false
local rem = v.removes
fallingblocks[i] = nil
if rem then
fakeblocktimer = fakeblockmaxtime
end
elseif v.die and v.y >= gridh+1 then --offset, MURDER IT!!!
gridwait[v.x] = false
fallingblocks[i] = nil
end
end
spawntime = spawntime - dt
if spawntime <= 0 then
spawntime = math.random(minspawntime, maxspawntime)
spawnblock()
end
end
function love.draw()
if fakeblocktimer > 0 then
love.graphics.push()
love.graphics.translate(0, recsize-fakeblocktimer/fakeblockmaxtime*recsize)
end
for x = 1, gridw do
for y = 1, gridh do
if grid[x][y] then
love.graphics.setColor(unpack(grid[x][y]))
love.graphics.rectangle("fill", (x-1)*recsize, (y-1)*recsize, recsize, recsize)
if pyramid then --TOOK ME A FREAKIN LONG TIME TO MAKE!
local p1 = {(x-1)*recsize, (y-1)*recsize,
(x-1)*recsize+recsize, (y-1)*recsize+recsize}
local p2 = {(x-1)*recsize+recsize/4, (y-1)*recsize+recsize/4,
(x-1)*recsize+recsize-recsize/4, (y-1)*recsize+recsize-recsize/4}
love.graphics.setColor(255, 255, 255, 55) --left
love.graphics.polygon("fill", p1[1], p1[2], p1[1], p1[4], p2[1], p2[4], p2[1], p2[2])
love.graphics.setColor(255, 255, 255, 75) --top
love.graphics.polygon("fill", p1[1], p1[2], p1[3], p1[2], p2[3], p2[2], p2[1], p2[2])
love.graphics.setColor(0, 0, 0, 55) --right
love.graphics.polygon("fill", p1[3], p1[2], p1[3], p1[4], p2[3], p2[4], p2[3], p2[2])
love.graphics.setColor(0, 0, 0, 75) --bottom
love.graphics.polygon("fill", p1[1], p1[4], p1[3], p1[4], p2[3], p2[4], p2[1], p2[4])
end
if not economic then
love.graphics.setColor(dark(grid[x][y]))
love.graphics.rectangle("fill", (x-1)*recsize, (y-1)*recsize, 2, recsize)
love.graphics.rectangle("fill", x*recsize-2, (y-1)*recsize, 2, recsize)
love.graphics.rectangle("fill", (x-1)*recsize+2, (y-1)*recsize, recsize-4, 2)
love.graphics.rectangle("fill", (x-1)*recsize+2, y*recsize-2, recsize-4, 2)
end
end
end
end
for i, v in pairs(fallingblocks) do
love.graphics.setColor(v.color)
love.graphics.rectangle("fill", (v.x-1)*recsize, (v.y-1)*recsize, recsize, recsize)
if pyramid then --TOOK ME A FREAKIN LONG TIME TO MAKE!
local p1 = {(v.x-1)*recsize, (v.y-1)*recsize,
(v.x-1)*recsize+recsize, (v.y-1)*recsize+recsize}
local p2 = {(v.x-1)*recsize+recsize/4, (v.y-1)*recsize+recsize/4,
(v.x-1)*recsize+recsize-recsize/4, (v.y-1)*recsize+recsize-recsize/4}
love.graphics.setColor(255, 255, 255, 55) --left
love.graphics.polygon("fill", p1[1], p1[2], p1[1], p1[4], p2[1], p2[4], p2[1], p2[2])
love.graphics.setColor(255, 255, 255, 75) --top
love.graphics.polygon("fill", p1[1], p1[2], p1[3], p1[2], p2[3], p2[2], p2[1], p2[2])
love.graphics.setColor(0, 0, 0, 55) --right
love.graphics.polygon("fill", p1[3], p1[2], p1[3], p1[4], p2[3], p2[4], p2[3], p2[2])
love.graphics.setColor(0, 0, 0, 75) --bottom
love.graphics.polygon("fill", p1[1], p1[4], p1[3], p1[4], p2[3], p2[4], p2[1], p2[4])
end
if not economic then
love.graphics.setColor(dark(v.color))
love.graphics.rectangle("fill", (v.x-1)*recsize, (v.y-1)*recsize, 2, recsize)
love.graphics.rectangle("fill", v.x*recsize-2, (v.y-1)*recsize, 2, recsize)
love.graphics.rectangle("fill", (v.x-1)*recsize+2, (v.y-1)*recsize, recsize-4, 2)
love.graphics.rectangle("fill", (v.x-1)*recsize+2, v.y*recsize-2, recsize-4, 2)
end
end
if fakeblocktimer > 0 then
love.graphics.pop()
end
love.graphics.setColor(0, 205, 255, 25)
local x, y = love.mouse.getPosition()
x = math.max(1, math.min(gridw, math.floor(x/recsize)+1))
y = math.max(1, math.min(gridw, math.floor(y/recsize)+1))
love.graphics.rectangle("fill", (x-1)*recsize, (y-1)*recsize, recsize, recsize)
love.graphics.setColor(205, 255, 0, 55)
love.graphics.line(0, maxheight*recsize, gridw*recsize, maxheight*recsize)
end
function love.keypressed(key, u)
if key == "enter" or key == "return" or key == "kpenter" then
economic = not economic
elseif key == "p" then
pyramid = not pyramid
elseif key == " " then
spawnblock()
elseif key == "r" then
for x = 1, gridw do
for y = 1, gridh do
if grid[x][y] then
grid[x][y] = {math.random(155, 255), math.random(155, 255), math.random(155, 255), 255}
end
end
end
for i, v in pairs(fallingblocks) do
v.color = {math.random(155, 255), math.random(155, 255), math.random(155, 255), 255}
end
elseif key == "down" or key == "s" or key == "delete" then
if fakeblocktimer == 0 then
for x = 1, gridw do --check if it CAN remove line
if grid[x][gridh] then
fakeblocktimer = fakeblockmaxtime
break
end
end
end
elseif key == "escape" then
love.event.quit()
end
end
function love.mousepressed(x, y, button)
if button == "wu" then
if simspeed < 1 then
simspeed = math.min(1, simspeed+.1)
else
simspeed = math.min(5, simspeed+1)
end
elseif button == "wd" then
if simspeed > 1 then
simspeed = math.max(1, simspeed-1)
else
simspeed = math.max(.1, simspeed-.1)
end
elseif button == "m" then
simspeed = 1
elseif button == "l" then
spawnblock(math.max(1, math.min(gridw, math.floor(x/recsize)+1)))
elseif button == "r" then
local rem = 0
if fakeblocktimer > 0 then
rem = 1-fakeblocktimer/fakeblockmaxtime
end
local nx = math.max(1, math.min(gridw, math.floor(x/recsize)+1))
local found = false
for i, v in pairs(fallingblocks) do
if v.x == nx and (v.y-1+rem)*recsize <= y and (v.y-1+rem)*recsize+recsize > y then --It should be this one!
v.color = {math.random(155, 255), math.random(155, 255), math.random(155, 255), 255}
found = true
break
end
end
if not found then --check stacked blocks
local ny = math.max(1, math.min(gridh, math.floor((y-(rem*recsize))/recsize)+1))
if grid[nx][ny] then
grid[nx][ny] = {math.random(155, 255), math.random(155, 255), math.random(155, 255), 255}
end
end
end
end
function spawnblock(prex)
local prex = prex or false
local xtable = {}
for x = 1, gridw do
if grid[x][maxheight] == false and not gridwait[x] and (prex == false or x == prex) then
table.insert(xtable, x)
end
end
if #xtable == 0 then
return --mostly won't happen, but just in case...
end
local x = xtable[math.random(#xtable)]
local dy = gridh
local removes = false
for y = 1, gridh do
if grid[x][y] == false then
dy = y
end
end
if dy == maxheight then
removes = true --removes last line of blocks
end
gridwait[x] = true
local col = {math.random(155, 255), math.random(155, 255), math.random(155, 255), 255}
local ft = {x = x, y = -1, dy = dy, color = col, removes = removes, speed = math.random(minspeed, maxspeed), die = false}
table.insert(fallingblocks, ft)
end
function removeline()
for i, v in pairs(fallingblocks) do
if v.dy < gridh then
v.dy = v.dy + 1
end
if v.y >= gridh-.75 then -- Almost falling? Let it fall!
v.die = true
end
v.y = v.y + 1
end
for x = 1, gridw do
for y = gridh, 2, -1 do
grid[x][y] = grid[x][y-1]
end
grid[x][1] = false
end
end
function dark(c)
local r, g, b, a = unpack(c)
return math.max(r*.75, 0), math.max(g*.75, 0), math.max(b*.75, 0), a
end
EDIT: Just like my last doodle, I made some improvements in this one. Now, by pressing "F", you can change the block's shapes (blocks, circles, hexagons or triangles). The new code:
Code: Select all
-- LÖVE Code Doodle #3
-- by HugoBDesigner
function love.load()
-- GOOD VARIABLE S TO MESS AROUND WITH:
recsize = 40 --Size, in pixels, of the blocks
maxheight = 12 --The maximum height of a pile of blocks
fakeblockmaxtime = 2 --The time it takes to remove a line after it surpasses the maximum height
gridw = math.floor(800/recsize)
gridh = math.floor(600/recsize)
maxheight = gridh-maxheight
fakeblocks = {}
fakeblocktimer = 0
simspeed = 1
grid = {}
gridwait = {}
minspawntime = .05
maxspawntime = .25
minspeed = 3
maxspeed = 12
spawntime = math.random(minspawntime, maxspawntime)
for x = 1, gridw do
grid[x] = {}
gridwait[x] = false
for y = 1, gridh do
grid[x][y] = false
end
end
economic = false
pyramid = true
fallingblocks = {}
forms = {"rectangle", "circle", "hexagon", "triangle"}
formn = 1
love.graphics.setBackgroundColor(255, 255, 255, 255)
end
function love.update(ndt)
local dt = ndt*simspeed
if fakeblocktimer > 0 then
fakeblocktimer = fakeblocktimer - dt
if fakeblocktimer <= 0 then
fakeblocktimer = 0
removeline()
end
return
end
for i, v in pairs(fallingblocks) do
v.y = v.y + v.speed*dt
if v.y >= v.dy and not v.die then
grid[v.x][v.dy] = v.color
gridwait[v.x] = false
local rem = v.removes
fallingblocks[i] = nil
if rem then
fakeblocktimer = fakeblockmaxtime
end
elseif v.die and v.y >= gridh+1 then --offset, MURDER IT!!!
gridwait[v.x] = false
fallingblocks[i] = nil
end
end
spawntime = spawntime - dt
if spawntime <= 0 then
spawntime = math.random(minspawntime, maxspawntime)
spawnblock()
end
end
function love.draw()
if fakeblocktimer > 0 then
love.graphics.push()
love.graphics.translate(0, recsize-fakeblocktimer/fakeblockmaxtime*recsize)
end
local drawt = {}
for x = 1, gridw do
for y = 1, gridh do
if grid[x][y] then
table.insert(drawt, {x = x, y = y, color = grid[x][y]})
end
end
end
for i, v in pairs(fallingblocks) do
table.insert(drawt, {x = v.x, y = v.y, color = v.color})
end
for i, v in pairs(drawt) do
love.graphics.setColor(unpack(v.color))
local f = forms[formn]
local px = {}
local ip = {}
if f == "hexagon" then
for i = 1, 6 do
table.insert(px, (v.x-1)*recsize+recsize/2+math.cos((math.pi*2)*((i-1)/6))*(recsize/2))
table.insert(px, (v.y-1)*recsize+recsize/2+math.sin((math.pi*2)*((i-1)/6))*(recsize/2))
table.insert(ip, (v.x-1)*recsize+recsize/2+math.cos((math.pi*2)*((i-1)/6))*(recsize/4))
table.insert(ip, (v.y-1)*recsize+recsize/2+math.sin((math.pi*2)*((i-1)/6))*(recsize/4))
end
end
if f == "rectangle" then
love.graphics.rectangle("fill", (v.x-1)*recsize, (v.y-1)*recsize, recsize, recsize)
if pyramid then --TOOK ME A FREAKIN LONG TIME TO MAKE!
local p1 = {(v.x-1)*recsize, (v.y-1)*recsize,
(v.x-1)*recsize+recsize, (v.y-1)*recsize+recsize}
local p2 = {(v.x-1)*recsize+recsize/4, (v.y-1)*recsize+recsize/4,
(v.x-1)*recsize+recsize-recsize/4, (v.y-1)*recsize+recsize-recsize/4}
love.graphics.setColor(255, 255, 255, 55) --left
love.graphics.polygon("fill", p1[1], p1[2], p1[1], p1[4], p2[1], p2[4], p2[1], p2[2])
love.graphics.setColor(255, 255, 255, 75) --top
love.graphics.polygon("fill", p1[1], p1[2], p1[3], p1[2], p2[3], p2[2], p2[1], p2[2])
love.graphics.setColor(0, 0, 0, 55) --right
love.graphics.polygon("fill", p1[3], p1[2], p1[3], p1[4], p2[3], p2[4], p2[3], p2[2])
love.graphics.setColor(0, 0, 0, 75) --bottom
love.graphics.polygon("fill", p1[1], p1[4], p1[3], p1[4], p2[3], p2[4], p2[1], p2[4])
end
elseif f == "circle" then
love.graphics.circle("fill", (v.x-1)*recsize+recsize/2, (v.y-1)*recsize+recsize/2, recsize/2, 32)
if pyramid then
love.graphics.setColor(255, 255, 255, 75)
love.graphics.circle("fill", (v.x-1)*recsize+recsize/2, (v.y-1)*recsize+recsize/2, recsize/2, 32)
love.graphics.setColor(unpack(v.color))
love.graphics.circle("fill", (v.x-1)*recsize+recsize/2, (v.y-1)*recsize+recsize/2, recsize/4, 32)
end
elseif f == "hexagon" then
love.graphics.polygon("fill", px)
if pyramid then
love.graphics.setColor(0, 0, 0, 55) --bottom
love.graphics.polygon("fill", px[5], px[6], px[3], px[4], ip[3], ip[4], ip[5], ip[6])
love.graphics.setColor(0, 0, 0, 75) --left-bottom
love.graphics.polygon("fill", px[3], px[4], px[1], px[2], ip[1], ip[2], ip[3], ip[4])
love.graphics.setColor(0, 0, 0, 55) --left-top
love.graphics.polygon("fill", px[1], px[2], px[11], px[12], ip[11], ip[12], ip[1], ip[2])
love.graphics.setColor(255, 255, 255, 55) --top
love.graphics.polygon("fill", px[9], px[10], px[11], px[12], ip[11], ip[12], ip[9], ip[10])
love.graphics.setColor(255, 255, 255, 75) --right-top
love.graphics.polygon("fill", px[7], px[8], px[9], px[10], ip[9], ip[10], ip[7], ip[8])
love.graphics.setColor(255, 255, 255, 55) --right-bottom
love.graphics.polygon("fill", px[7], px[8], px[5], px[6], ip[5], ip[6], ip[7], ip[8])
end
elseif f == "triangle" then
local px = {(v.x-1)*recsize+recsize/2, (v.x-1)*recsize, (v.x-1)*recsize+recsize}
local py = {(v.y-1)*recsize, (v.y-1)*recsize+recsize, (v.y-1)*recsize+recsize}
local cx = {(v.x-1)*recsize+recsize/2, (v.x-1)*recsize+recsize/3, (v.x-1)*recsize+recsize/3*2}
local cy = {(v.y-1)*recsize+recsize/3, (v.y-1)*recsize+recsize/4*3, (v.y-1)*recsize+recsize/4*3}
love.graphics.polygon("fill", px[1], py[1], px[2], py[2], px[3], py[3])
if pyramid then
love.graphics.setColor(255, 255, 255, 75) --top-left
love.graphics.polygon("fill", px[1], py[1], cx[1], cy[1], cx[2], cy[2], px[2], py[2])
love.graphics.setColor(0, 0, 0, 55) --top-right
love.graphics.polygon("fill", px[1], py[1], cx[1], cy[1], cx[3], cy[3], px[3], py[3])
love.graphics.setColor(0, 0, 0, 75) --bottom
love.graphics.polygon("fill", px[2], py[2], cx[2], cy[2], cx[3], cy[3], px[3], py[3])
end
end
if not economic then
love.graphics.setColor(dark(v.color))
if f == "rectangle" then
love.graphics.rectangle("fill", (v.x-1)*recsize, (v.y-1)*recsize, 2, recsize)
love.graphics.rectangle("fill", v.x*recsize-2, (v.y-1)*recsize, 2, recsize)
love.graphics.rectangle("fill", (v.x-1)*recsize+2, (v.y-1)*recsize, recsize-4, 2)
love.graphics.rectangle("fill", (v.x-1)*recsize+2, v.y*recsize-2, recsize-4, 2)
elseif f == "circle" then
love.graphics.circle("line", (v.x-1)*recsize+recsize/2, (v.y-1)*recsize+recsize/2, recsize/2, 32)
elseif f == "hexagon" then
love.graphics.polygon("line", px)
elseif f == "triangle" then
local px = {(v.x-1)*recsize+recsize/2, (v.x-1)*recsize, (v.x-1)*recsize+recsize}
local py = {(v.y-1)*recsize, (v.y-1)*recsize+recsize, (v.y-1)*recsize+recsize}
love.graphics.polygon("line", px[1], py[1], px[2], py[2], px[3], py[3])
end
end
end
if fakeblocktimer > 0 then
love.graphics.pop()
end
love.graphics.setColor(0, 205, 255, 25)
local x, y = love.mouse.getPosition()
x = math.max(1, math.min(gridw, math.floor(x/recsize)+1))
y = math.max(1, math.min(gridw, math.floor(y/recsize)+1))
love.graphics.rectangle("fill", (x-1)*recsize, (y-1)*recsize, recsize, recsize)
love.graphics.setColor(205, 255, 0, 55)
love.graphics.line(0, maxheight*recsize, gridw*recsize, maxheight*recsize)
end
function love.keypressed(key, u)
if key == "enter" or key == "return" or key == "kpenter" then
economic = not economic
elseif key == "p" then
pyramid = not pyramid
elseif key == " " then
spawnblock()
elseif key == "r" then
for x = 1, gridw do
for y = 1, gridh do
if grid[x][y] then
grid[x][y] = {math.random(155, 255), math.random(155, 255), math.random(155, 255), 255}
end
end
end
for i, v in pairs(fallingblocks) do
v.color = {math.random(155, 255), math.random(155, 255), math.random(155, 255), 255}
end
elseif key == "down" or key == "s" or key == "delete" then
if fakeblocktimer == 0 then
for x = 1, gridw do --check if it CAN remove line
if grid[x][gridh] then
fakeblocktimer = fakeblockmaxtime
break
end
end
end
elseif key == "escape" then
love.event.quit()
elseif key == "f" then
formn = formn+1
if formn > #forms then
formn = 1
end
end
end
function love.mousepressed(x, y, button)
if button == "wu" then
if simspeed < 1 then
simspeed = math.min(1, simspeed+.1)
else
simspeed = math.min(5, simspeed+1)
end
elseif button == "wd" then
if simspeed > 1 then
simspeed = math.max(1, simspeed-1)
else
simspeed = math.max(.1, simspeed-.1)
end
elseif button == "m" then
simspeed = 1
elseif button == "l" then
spawnblock(math.max(1, math.min(gridw, math.floor(x/recsize)+1)))
elseif button == "r" then
local rem = 0
if fakeblocktimer > 0 then
rem = 1-fakeblocktimer/fakeblockmaxtime
end
local nx = math.max(1, math.min(gridw, math.floor(x/recsize)+1))
local found = false
for i, v in pairs(fallingblocks) do
if v.x == nx and (v.y-1+rem)*recsize <= y and (v.y-1+rem)*recsize+recsize > y then --It should be this one!
v.color = {math.random(155, 255), math.random(155, 255), math.random(155, 255), 255}
found = true
break
end
end
if not found then --check stacked blocks
local ny = math.max(1, math.min(gridh, math.floor((y-(rem*recsize))/recsize)+1))
if grid[nx][ny] then
grid[nx][ny] = {math.random(155, 255), math.random(155, 255), math.random(155, 255), 255}
end
end
end
end
function spawnblock(prex)
local prex = prex or false
local xtable = {}
for x = 1, gridw do
if grid[x][maxheight] == false and not gridwait[x] and (prex == false or x == prex) then
table.insert(xtable, x)
end
end
if #xtable == 0 then
return --mostly won't happen, but just in case...
end
local x = xtable[math.random(#xtable)]
local dy = gridh
local removes = false
for y = 1, gridh do
if grid[x][y] == false then
dy = y
end
end
if dy == maxheight then
removes = true --removes last line of blocks
end
gridwait[x] = true
local col = {math.random(155, 255), math.random(155, 255), math.random(155, 255), 255}
local ft = {x = x, y = -1, dy = dy, color = col, removes = removes, speed = math.random(minspeed, maxspeed), die = false}
table.insert(fallingblocks, ft)
end
function removeline()
for i, v in pairs(fallingblocks) do
if v.dy < gridh then
v.dy = v.dy + 1
end
if v.y >= gridh-.75 then -- Almost falling? Let it fall!
v.die = true
end
v.y = v.y + 1
end
for x = 1, gridw do
for y = gridh, 2, -1 do
grid[x][y] = grid[x][y-1]
end
grid[x][1] = false
end
end
function dark(c)
local r, g, b, a = unpack(c)
return math.max(r*.75, 0), math.max(g*.75, 0), math.max(b*.75, 0), a
end
- HugoBDesigner
- Party member
- Posts: 403
- Joined: Mon Feb 24, 2014 6:54 pm
- Location: Above the Pocket Dimension
- Contact:
Re: Code Doodles!
Two doodles in a row!!!
First time I make something 3D-ish that works! Here comes the keys!
Enter: Toggles wireframe mode
Space/"R": Randomizes cube and background colors
F12: Toggles mouse grab
"-": Decreases cube size
"+": Increases cube size
Mouse wheel: Increases/Decreases cube depth
And the code:
First time I make something 3D-ish that works! Here comes the keys!
Enter: Toggles wireframe mode
Space/"R": Randomizes cube and background colors
F12: Toggles mouse grab
"-": Decreases cube size
"+": Increases cube size
Mouse wheel: Increases/Decreases cube depth
And the code:
Code: Select all
-- LÖVE Code Doodle #4
-- by HugoBDesigner
function love.load()
love.mouse.setGrab = love.mouse.setGrab or love.mouse.setGrabbed
depth = 70
rec = 100
x1 = 0
y1 = 0
mult = 1
mode = true
love.graphics.setLineWidth(2)
cubecolor = {}
backcolor = {}
randomColors()
end
function love.update(dt)
x1, y1 = love.mouse.getPosition()
x1 = x1-400
y1 = y1-300
end
function love.draw()
local px1, py1 = x1-rec/2, y1-rec/2
local px2, py2 = x1-rec/2+rec, y1-rec/2+rec
local bx1, by1 = px1+rec/2-depth*(x1/800)*mult-rec/100*depth/2, py1+rec/2-depth*(y1/600)*mult-rec/100*depth/2
local bx2, by2 = bx1+rec/100*depth, by1+rec/100*depth
love.graphics.push()
love.graphics.translate(400, 300)
local r, g, b, a = unpack(cubecolor)
love.graphics.setColor(r*.5, g*.5, b*.5, a)
if mode then love.graphics.rectangle("fill", bx1, by1, rec/100*depth, rec/100*depth) end --back
love.graphics.setColor(r*.15, g*.15, b*.15, a)
love.graphics.rectangle("line", bx1, by1, rec/100*depth, rec/100*depth)
love.graphics.setColor(r*.75, g*.75, b*.75, a)
if by1 <= py1 or mode == false then --top
if mode then love.graphics.polygon("fill", px1, py1, bx1, by1, bx2, by1, px2, py1) end
love.graphics.setColor(r*.15, g*.15, b*.15, a)
love.graphics.polygon("line", px1, py1, bx1, by1, bx2, by1, px2, py1)
end
if by2 >= py2 or mode == false then --bottom
if mode then love.graphics.polygon("fill", px1, py2, bx1, by2, bx2, by2, px2, py2) end
love.graphics.setColor(r*.15, g*.15, b*.15, a)
love.graphics.polygon("line", px1, py2, bx1, by2, bx2, by2, px2, py2)
end
love.graphics.setColor(r*.5, g*.5, b*.5, a)
if bx1 <= px1 or mode == false then --left
if mode then love.graphics.polygon("fill", px1, py1, bx1, by1, bx1, by2, px1, py2) end
love.graphics.setColor(r*.15, g*.15, b*.15, a)
love.graphics.polygon("line", px1, py1, bx1, by1, bx1, by2, px1, py2)
end
if bx2 >= px2 or mode == false then --right
if mode then love.graphics.polygon("fill", px2, py1, bx2, by1, bx2, by2, px2, py2) end
love.graphics.setColor(r*.15, g*.15, b*.15, a)
love.graphics.polygon("line", px2, py1, bx2, by1, bx2, by2, px2, py2)
end
love.graphics.setColor(r, g, b, a)
if mode then love.graphics.rectangle("fill", px1, py1, rec, rec) end --front
love.graphics.setColor(r*.15, g*.15, b*.15, a)
love.graphics.rectangle("line", px1, py1, rec, rec)
love.graphics.pop()
love.graphics.setColor(255, 255, 255, 255)
love.graphics.print("FPS = "..love.timer.getFPS(), 4, 4)
love.graphics.print("(Mouse Wheel) Depth = "..mult, 4, 20)
love.graphics.print("(+/-) Cube size = "..rec, 4, 36)
love.graphics.print("(Enter) Wireframe mode = "..tostring(not mode), 4, 52)
love.graphics.print("(Space/R) Randomizes colors", 4, 68)
love.graphics.print("(F12) " .. (love.mouse.isGrabbed() and "Ungrab" or "Grab") .. " mouse", 4, 84)
end
function love.keypressed(k, u)
if k == "escape" then
love.event.quit()
elseif k == "enter" or k == "return" or k == "kpenter" then
mode = not mode
elseif k == "f12" then
love.mouse.setGrab(not love.mouse.isGrabbed())
elseif k == "-" then
rec = math.max(50, rec-10)
elseif k == "=" then
rec = math.min(300, rec+10)
elseif k == "r" or k == " " then
randomColors()
end
end
function love.mousepressed(x, y, b)
if b == "wd" then
mult = math.max(mult-.2, .5)
elseif b == "wu" then
mult = math.min(mult+.2, 6)
end
end
function randomColors()
cubecolor = {math.random(155, 255), math.random(155, 255), math.random(155, 255), 255}
backcolor = {math.random(0, 100), math.random(0, 100), math.random(0, 100), 255}
love.graphics.setBackgroundColor(unpack(backcolor))
end
Re: Code Doodles!
This doodle is kind of a happy accident. At least some aspects of it. Basically i was gonna mix some shaders with it, So i set up a couple canvases, Forgot to clear one of them, And it ended up looking neat.
the code is mad dirty.

Code: Select all
screen = {
width = love.graphics.getWidth(),
height = love.graphics.getHeight()
}
love.window.setMode(screen.width, screen.height, {fsaa = 4})
local objects = {}
local radius = 12.5
local width = math.floor(screen.width / radius)
local height = math.floor(screen.height / radius)
local color = {0, 255, 126}
local color_speed = 0.01
local canvas = {
love.graphics.newCanvas(screen.width, screen.height),
love.graphics.newCanvas(screen.width, screen.height)
}
local light = {
x = 0,
y = 0,
size = 32
}
function love.load()
local sx = radius
local sy = radius
local cx = sx
local cy = sy
for y=0, height-1 do
for x=0, height-1 do
objects[#objects + 1] = {
x = cx,
y = cy,
radius = radius,
alpha = 1,
color = color
}
cx = cx + (radius * 2)
end
cx = sx
cy = cy + (radius * 2)
end
end
function love.update(dt)
local mx, my = love.mouse.getPosition()
light.x = light.x + (mx - light.x) * 0.1
light.y = light.y + (my- light.y) * 0.1
for i,v in ipairs(objects) do
local dist = getDistance(light.x, light.y, v.x, v.y)
v.alpha = 1 / (dist / light.size)
if v.alpha > 1 then v.alpha = 1 end
v.color[1] = v.color[1] + color_speed * dt
if v.color[1] > 255 then v.color[1] = 0 end
end
end
function love.draw()
love.graphics.setColor(255, 255, 255, 255)
canvas[1]:clear()
love.graphics.setCanvas(canvas[1])
for i,v in ipairs(objects) do
love.graphics.setColor(hsl(v.color[1], v.color[2], v.color[3], 128 * v.alpha))
love.graphics.rectangle("fill", v.x - (v.radius * v.alpha), v.y - (v.radius * v.alpha), (v.radius * 2) * v.alpha, (v.radius * 2) * v.alpha)
love.graphics.setColor(hsl(v.color[1], v.color[2], v.color[3], 255 * v.alpha))
love.graphics.rectangle("line", v.x - (v.radius * v.alpha), v.y - (v.radius * v.alpha), (v.radius * 2) * v.alpha, (v.radius * 2) * v.alpha)
end
love.graphics.setCanvas(canvas[2])
--shader
love.graphics.setColor(255, 255, 255, 255)
love.graphics.draw(canvas[1])
love.graphics.setCanvas()
--Final drawing
love.graphics.draw(canvas[2])
end
function love.keypressed(key)
if key == "escape" then love.event.push("quit") end
end
function getDistance(x1,y1, x2,y2)
return ((x2-x1)^2+(y2-y1)^2)^0.5
end
function hsl(h, s, l, a)
if s<=0 then return l,l,l,a end
h, s, l = h/256*6, s/255, l/255
local c = (1-math.abs(2*l-1))*s
local x = (1-math.abs(h%2-1))*c
local m,r,g,b = (l-.5*c), 0,0,0
if h < 1 then r,g,b = c,x,0
elseif h < 2 then r,g,b = x,c,0
elseif h < 3 then r,g,b = 0,c,x
elseif h < 4 then r,g,b = 0,x,c
elseif h < 5 then r,g,b = x,0,c
else r,g,b = c,0,x
end return (r+m)*255,(g+m)*255,(b+m)*255,a
end

Re: Code Doodles!
Just a look-see what it would take to implement a button capability.
- Attachments
-
button_button.love
- Simple button test
- (26.06 KiB) Downloaded 316 times
- CaptainMaelstrom
- Party member
- Posts: 161
- Joined: Sat Jan 05, 2013 10:38 pm
Re: Code Doodles!
I made a little code doodle a few days ago. This thread is awesome for getting the creative juices flowing.
I'm attaching the .love, but I'd really appreciate it if somebody could tell me how to make a copy I can distribute to my friends who use OS X. I tried following the "Creating a Mac OS X App" section here: http://www.love2d.org/wiki/Game_Distribution . But my friend said when he tried to run it, he gets the "no game" screen. Ideas?
I'm attaching the .love, but I'd really appreciate it if somebody could tell me how to make a copy I can distribute to my friends who use OS X. I tried following the "Creating a Mac OS X App" section here: http://www.love2d.org/wiki/Game_Distribution . But my friend said when he tried to run it, he gets the "no game" screen. Ideas?
- Attachments
-
- midnight.osx.zip
- broken mac distribution : (
- (7.81 MiB) Downloaded 253 times
-
midnight.love
- The .love
- (4.1 MiB) Downloaded 386 times
Who is online
Users browsing this forum: No registered users and 3 guests