the way i've implemented it, if a brick is hit, a pre-initialized particle system is cloned and stored in a table for drawing/updating in the usual callbacks. the position is set to the position of the block that was hit, via setPosition(). in the update and draw process, update is called on each stored particlesystem, and the draw calls are made for each as usual.
What I'm seeing, is everything behaving normally, *except* that the particle emitter seems to be displayed doing its thing at some random coordinates down and to the right of the coordinates I have setPosition() on. upon examination of empty emitters prior to removal, their position is set as expected.
1. what might be going on here?
2. should I be cloning particle systems like that?
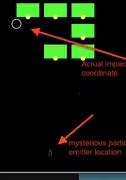