So anyways let's get started with the topic.
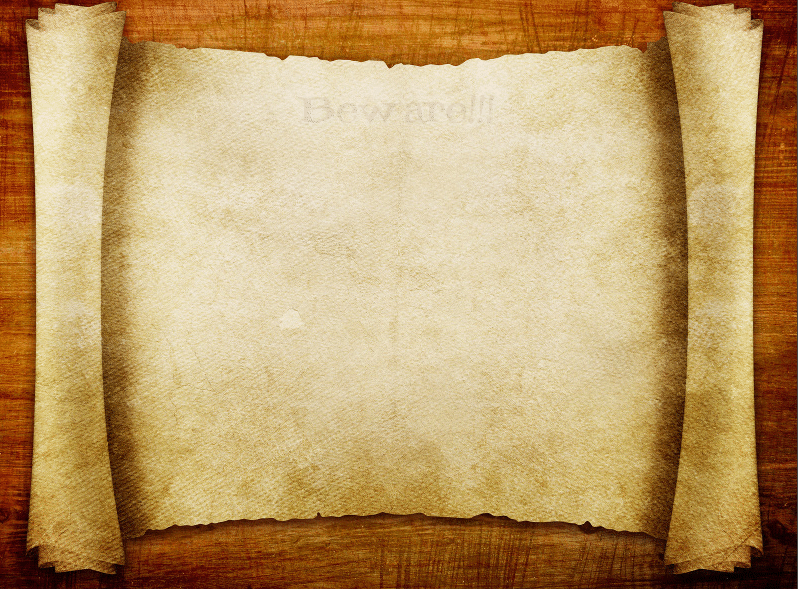
And if you didn't already figure it out then let me reveal that this animation was created in LOVE2D - not in some animation software. And no other library except Anima was used to create this animation. And the source was some 50 lines - almost all of it trivial stuff.
Enough show-off let's start the topic alreadyIf you can't follow with the tutorial (short documentation - whatever you want to call it) then visit https://github.com/YoungNeer/lovelib/tr ... ext%20Demo and see the code in the Demos to get an Idea how things work
Also if you want the link for Anima then here's it (although you can get it from the attachment which has a demo with it)
https://github.com/YoungNeer/lovelib/tree/master/Anima/
How can we make an awesome typing animation using Anima?
Step#0- The require Step:
Code: Select all
Anima=require 'Anima'
And then in love.load function, create something which I call an animation object, for eg.-
Code: Select all
typingAnim=Anima:init()
Code: Select all
typinganim:newTypingAnimation(text,speed) --load the animation
--text is string and speed is number (unit- letter per second)
typinganim:animationStart() --actually start the animation
Code: Select all
typingAnim:startNewTypingAnimation(text,speed) -- load and start the animation
Anyways that was init step. Now comes the update step
Step#2- The update Step:
In other context the update step can be very complicated but in our context where we have only one simple typing animation this step is pretty simple:-
Add this to love.update function
Code: Select all
typingAnim:update(dt)
Step#3- The draw Step:
Code: Select all
typingAnim:draw(x,y,...bla,bla)
---if you are simply drawing typing text
typingAnim:drawF(x,y,...bla,bla)
---when you are drawing FORMATTED typing text
Code: Select all
typingAnim:setTypingSpeed(speed)
Okay now let's get deeper!
I am sorry I lied to you in the beginning - both newTypingAnimation and startNewTypingAnimation accepts three parameters not two

And if that is not enough then you can also make your function more dynamic such as don't play *tic-tic* sound for space. Well how'd you do that? Simple- Anima will pass the key that was just printed as the first and argument in the function so you get to decide what to do for which key. Ex-
Code: Select all
function playTypingSound(char)
if char~=' ' then typingSound:play() end
end
Now finally some relatively complicated stuff

So what if you have an extra animation such as moving animation or scaling animation, etc. Well worry not instead of saying newTypingAnimation or startNewTypingAnimation you will simply say newAnimation or startNewAnimation. And both of these functions are kinda heavily overloaded so there's no generic format. But the one that you will use the most is:-
Code: Select all
typingAnim:newAnimation(operation,...)
Here operation is a string and ... depends on what that string is (I told you it's heavily overloaded). So let's briefly see the all possible options for this format:-
Code: Select all
typingAnima:newAnimation('move',x,y)
typingAnima:newAnimation('rotate',r)
typingAnima:newAnimation('scale',sx,sy)
typingAnima:newAnimation('opacity',a)
So if you want moving animation along with typing animation you'd simply do this in the init step:- (for eg.)
Code: Select all
typingAnim:newTypingAnimation("your text",2)
typingAnim:newAnimation('move',50)
-- y is defaulted to zero means no movement along vertical
typingAnim:setMoveSpeed(5)
-- if you don't explicitly set move speed then it's 1 by default
typingAnim:startAnimation()
--start the animation
And now comes the draw step, actually there's nothing complicated about draw - this will be the same as before.
So I was just joking the whole time
