I've been following Lode Vandavenne's excellent raycasting tutorial and am now trying to get floorcasting to work.
https://lodev.org/cgtutor/raycasting2.html
The only problem is, even after copying the code word for word (adjusting for lua syntax of course) multiple times, the end result always ends up with a terrible grainy look.
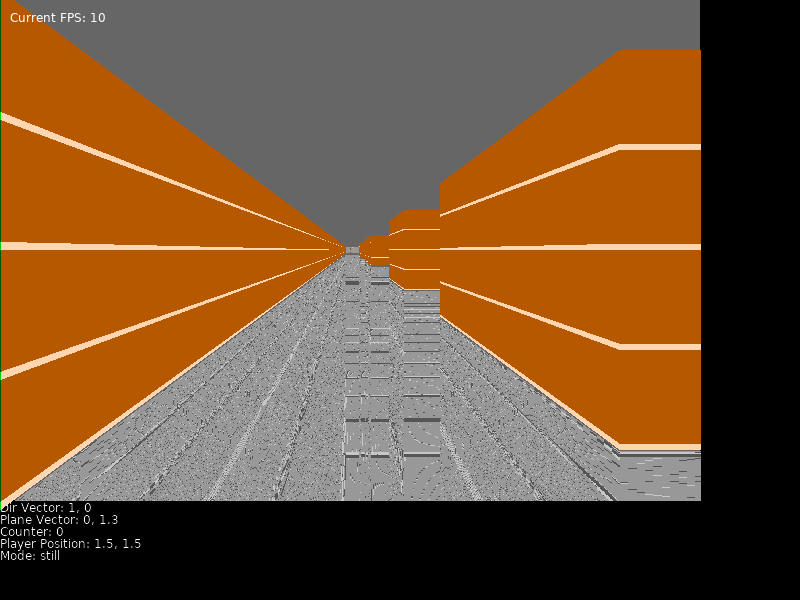
On top of this, the floor texture seems to be displaying wrong regardless of the grainy effect. The texture appears to display straight down rather than how it should given the perspective.
My code for floorcasting looks as such:
Code: Select all
-- Floor drawing...
local h = self.screenH
local floorXWall, floorYWall
if side == 0 then
if rayDirX > 0 then
floorXWall = mapX
elseif rayDirX < 0 then
floorXWall = mapX + 1.0
end
floorYWall = mapY + wallX
elseif side == 1 then
floorXWall = mapX + wallX
if rayDirY > 0 then
floorYWall = mapY
elseif rayDirY < 0 then
floorYWall = mapY + 1.0
end
end
local distWall, distPlayer, currentDist
distWall = perpWallDist
distPlayer = 0
if drawEnd > 10000 then drawEnd = h end
for y = drawEnd+1, h do
local currentDist = h / (2.0 * y - h)
local weight = (currentDist - distPlayer) / (distWall - distPlayer)
local currentFloorX = weight * floorXWall + (1.0 - weight) * self.posX;
local currentFloorY = weight * floorYWall + (1.0 - weight) * self.posY;
local floorTexX, floorTexY;
local texWidth, texHeight = self.textures[1]:getWidth(), self.textures[1]:getWidth()
floorTexX = round(currentFloorX * texWidth) % texWidth;
floorTexY = round(currentFloorY * texHeight) % texHeight;
self.textures[1]:drawPixel(floorTexX+1, floorTexY+1, math.floor(x), math.ceil(y))
end
Code: Select all
tex.pixels = {}
for col = 1, tex.image:getWidth() do
tex.pixels[col] = {}
for row = 1, tex.image:getHeight() do
table.insert(tex.pixels[col], love.graphics.newQuad(row, col, 1, 1, tex.image:getWidth(), tex.image:getHeight()))
--tex.pixels[#tex.pixels+1] = )
end
end


P.S. The code is extremely slow due to excessive use of quads, but I think I'll get around to fixing that when I actually get the code into a place where it works.
P.P.S. Sorry in advance for horribly ugly code. I think I've outlined the bits that are important and I've included a short text file explaining useful bits. Thanks again!