
I'm working on an isometric 3D kind of thing and I'm using L2D because I really want to get into the guts of my rendering, so it seems like a good choice.
Right now I'm having kind of a major problem with z-sorting of triangles. Everything loads in and renders properly, except when multiple triangles share a common average z value, like so:
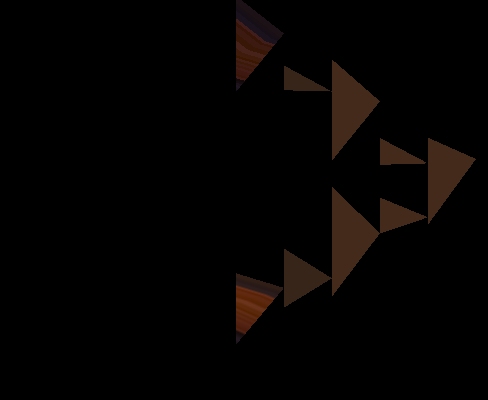
If no matching z values are found, like if the entire set is rotated from 2-44 degrees or if I offset the whole thing a teensy tiny bit right from the start so that it's .1 degree off the whole axis:
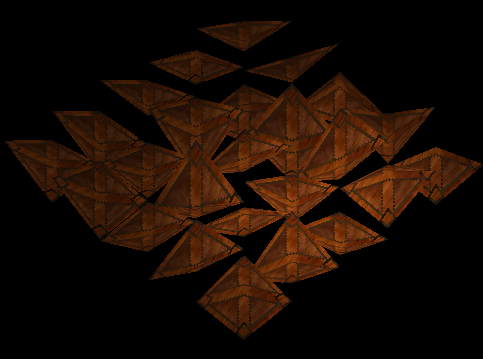
On some small level that kind of works for now, but I'm trying to avoid the whole problem down the line, since I expect I'm going to have a lot more and more complex geometry in the final thing than this test map does. Here's where I'm running into a problem:
Code: Select all
function zSortTriangles(object3D)
local sortedTable = {}
local pairsTable = {}
local zValueTable = {}
for i, tri in ipairs(object3D) do
local averageZ = 0
for _,vert in ipairs(tri) do
averageZ = averageZ + vert[3]
end
averageZ = averageZ / 3
if not pairsTable[averageZ] == nil then
table.insert(zValueTable,averageZ)
table.insert(pairsTable[averageZ],tri)
else
pairsTable[averageZ] = {}
table.insert(zValueTable,averageZ)
table.insert(pairsTable[averageZ],tri)
end
end
table.sort(zValueTable)
for i, val in ipairs(zValueTable) do
for i, tri in ipairs(pairsTable[val]) do
table.insert(sortedTable,tri)
end
end
return sortedTable
end
An object composed of un-ordered triangles is passed in, an object of the triangles sorted with the furthest back triangles first is passed out, and then each vertice of the set is broken down into something that can be handled by Mesh:setVertices() (A later step in code strips out the Z value from each vertex to fit the proper xy/uv/rgba format for a mesh.)
The problem seems to be with how I'm handling redundant results for the "averageZ" calculation for every triangle. It seems logical to use the result as an index, then sort those indices over a table and iterate it back, but there's something screwy with the results any time that actually happens.
The Mesh mode is set to ''triangles' too, so rendering order shouldn't matter, but my vertices are clearly getting screwed up somehow by this function and I have no idea what's causing them to get so jumbled.
Is there anything obvious in my code that I goofed?