---
I have an indexed png sprite. I would like to recolor that sprite in real time using a separate palette image.
Here's an example of what I'm trying to accomplish:
My sprite:


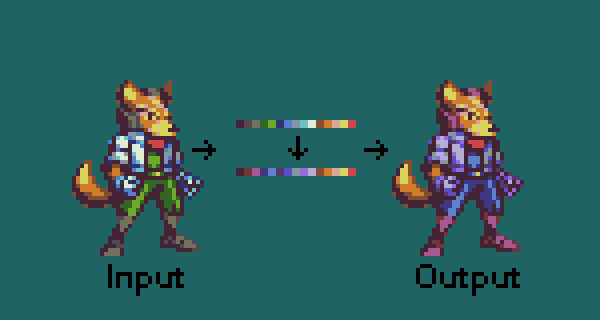
This seemed like a good place to use a shader, so I set about searching for one, or at least some guidelines for writing one.
Eventually, that brought me to this link:
https://www.khronos.org/opengl/wiki/Com ... d_textures
A paletted texture sounded like exactly what I was going for, so I attempted to convert their shader into something I could use in LÖVE.
This is what I came up with:
Code: Select all
//Fragment shader
uniform sampler2D ColorTable; // 16 x 1 pixels
uniform sampler2D MyIndexTexture;
varying vec2 TexCoord0;
vec4 effect( vec4 color, Image MyIndexTexture, vec2 TexCoord0, vec2 screen_coords ){
//What color do we want to index?
vec4 myindex = texture2D(MyIndexTexture, TexCoord0);
//Do a dependency texture read
vec4 texel = texture2D(ColorTable, myindex.xy);
return texel; //Output the color
}
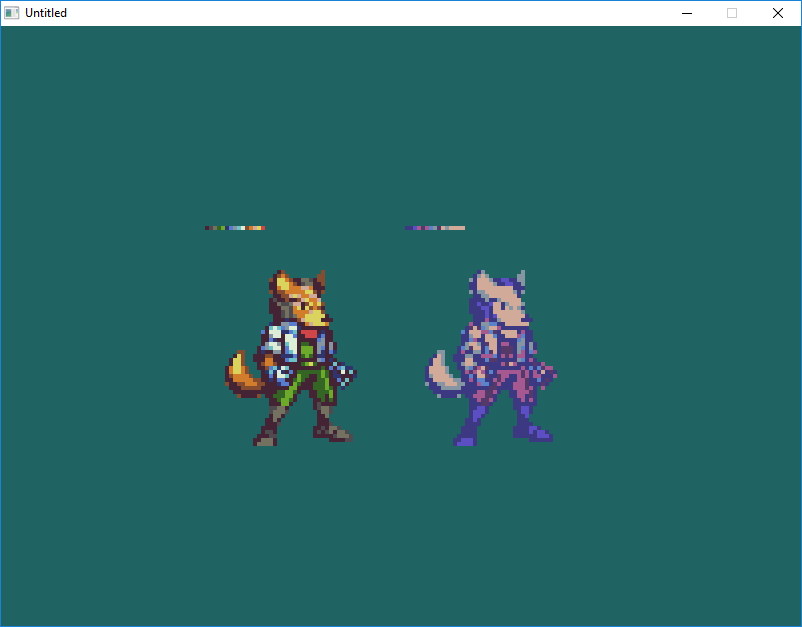
It's close, but some groups of colors are being set to a single color.
For example, the orange, yellow, pink and white hues on the original sprite are all being set to pink on the modified sprite.
Any help to fix this (or perhaps a different shader with a different methodology for accomplishing the same thing) would be greatly appreciated.
Things I have tried:
- Turning on gamma-correct rendering (just makes it worse)
Using a 256 x 1 palette (still converts multiple colors to the same color)
Using ImageData:mapPixel() and pre-rendering the sprites (useful, but too slow for real-time)
Finally, here is a simple .love illustrating the problem.