The fallowing code recreates that effect:
Code: Select all
-- Using love 0.10.x
love.graphics.setLineStyle "rough"
love.graphics.setDefaultFilter("nearest", "nearest")
love.window.setMode(512, 512, {borderless=true})
local rand, abs = love.math.random, math.abs
local mx, my = love.mouse.getX, love.mouse.getY
local screen = love.graphics.newCanvas(128,128)
local strength = 512 -- The more circles the stronger the effect.
function love.draw()
BEGIN_DRAW()
love.graphics.setColor(0xde, 0xad, 0x75)
love.graphics.circle("fill",mx()/4, my()/4,8)
END_DRAW()
end
function BEGIN_DRAW()
love.graphics.setCanvas(screen)
-- Set the background color
love.graphics.setColor(0x84, 0xe8, 0xd4)
--
-- The Magic happens here.
-- Repetitively draw small circles to the screen,
-- which clears parts of the previous frame,
-- thus creating creating a nice trailing effect
--
for i = 1, strength do
love.graphics.circle("line", rand(0,128), rand(0,128), 2)
end
love.graphics.setColor (0xff, 0xff, 0xff)
end
function END_DRAW() -- render frame.
love.graphics.setCanvas()
love.graphics.setColor (0xff, 0xff, 0xff)
love.graphics.draw(screen,0,0,0,4,4)
end
function love.keypressed(key, code, isrepeat)
if key == "escape" then love.event.quit() end
end
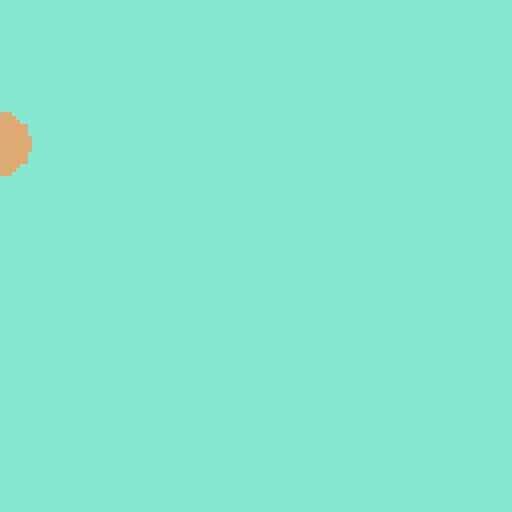
However, you're going to need to ask Trasevol about the other stuff :p