This game involves a world with game objects in a top down perspective. Rotation is applied to the view of the world.
I want to know if there is a better way to do what I am doing...
Currently I am doing this:
-> Instantiate a data structure that holds grid points as keys. Keys hold a set of values per key IE: "[0,0]" : {Game Object 1,2..11}
This data structure is a Set Map of grid point that are determined by the "CELL_SIZE" constant of my world.
For example, I take the X and Y of the game object and do CELL_X,CELLY = math.floor(x/CELL_SIZE),math.floor(y/CELL_SIZE)
-> Instantiate a data structure that holds grid points as keys, the difference between this object and the previous Set Map is that I rotate the game object position and THEN floor the x,y as grid cell coordinates. This makes sure that the cells in the Set Map aren't just the top left corner of the object. If it covers 6 cells, and it only gets placed into 1, the drawing execution won't know it's supposed to be one of those 6 cells to be drawn in, since it didn't get placed where it is telling the screen it is visible.
-> Instantiate Game object "GO"
-> Apply World_Position to "GO", place into the first Set Map.
The game object applies itself into the Set Map with a function that the user defines how the object overlaps into the grid space's cells based on offsets and such.
-> Apply rotated Screen_Position to "GO", place into the second Set Map.
Set Map #2 is used to be iterated over when drawing what is supposed to be in the screen. (I believe this is the most efficient way to find what should be in the screen without checking every single object's rotation, and then drawing)
Now, is it wise to iterate through everything on the screen and compile into a layer object and then draw all of the objects at once?
ISSUES-
#1 Is there another way to determine how many cells in the grid an object can cover with a polygon?
#2 If an object rotates at its position, how do I deal with that? lol
#3 Every time an object moves, it has to remove all instances of its position from the grid maps, and then place itself back in. (It doesn't really matter since my game won't involve constantly adding and removing game objects, but still.
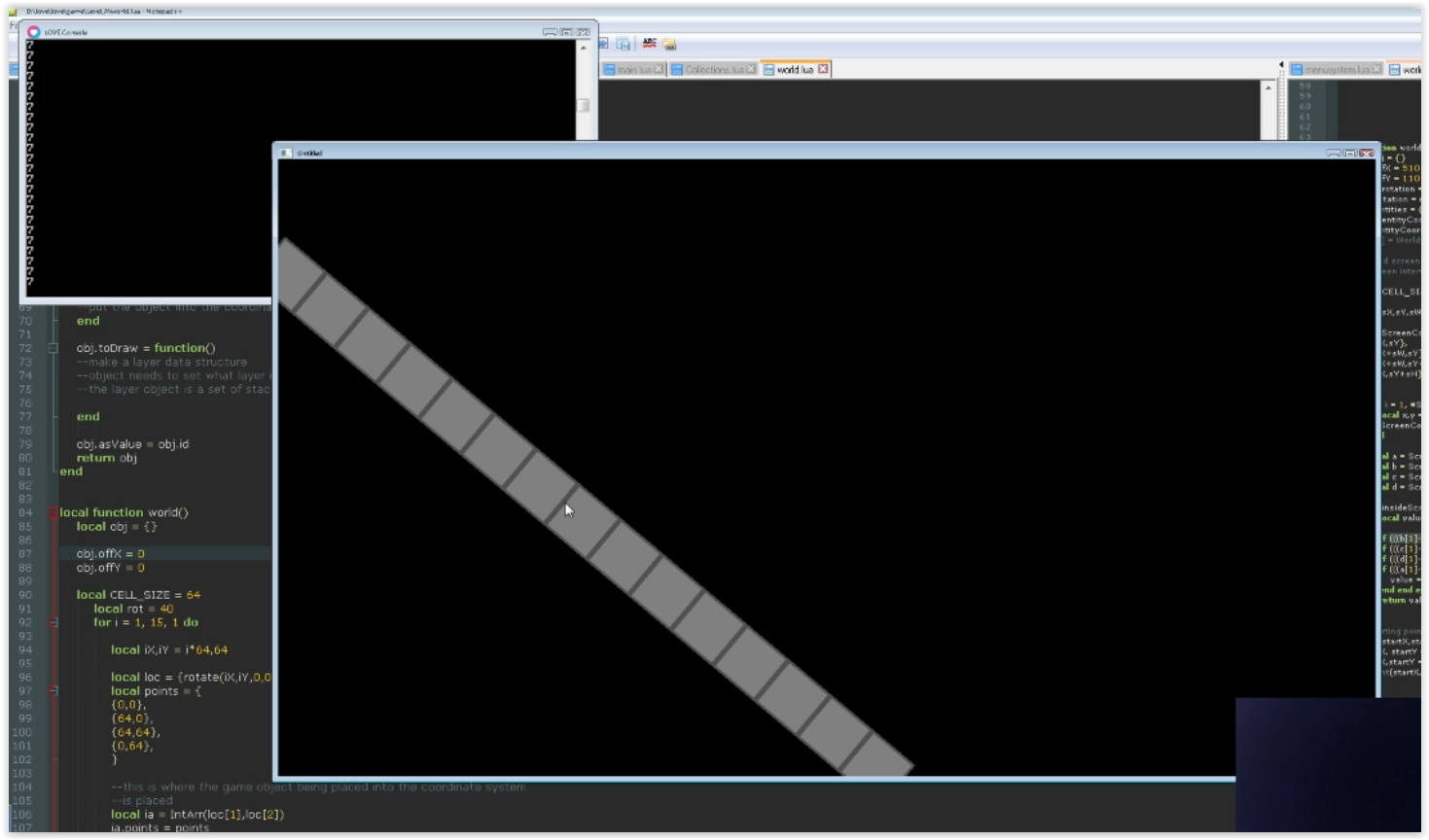
I have mouse targeting working 100%. It uses the object's hitbox to determine if it has been picked in the world.
It involves rotating in the opposite angle of the game screen rotation, and then using the non-rotated grid Set Map to loop over the objects in the cell that the mouse resides in. I do this so the game object hitbox doesn't have to perform rotation
pastebinned code
http://pastebin.com/rGGSuaEn
Am I headed in the right direction?