I am trying to make a new shader that splits up R-, G-, and B-channels into separate layers that converge, which will create an old-vcr-feel to it, like Hotline Miami has done.
The problem is that Lua Shader coding is very unintuitive.
This is the code I found in the Unity forums for a GLSL shader that does this:
Code: Select all
Shader "Custom/ChromaticAberration" {
Properties {
_MainTex ("Base (RGB)", 2D) = "white" {}
}
SubShader
{
Pass
{
CGPROGRAM
#pragma vertex vert_img
#pragma fragment frag
#pragma fragmentoption ARB_precision_hint_fastest
#include "UnityCG.cginc"
uniform sampler2D _MainTex;
uniform float _AberrationOffset;
float4 frag(v2f_img i) : COLOR
{
float2 coords = i.uv.xy;
_AberrationOffset /= 300.0f;
//Red Channel
float4 red = tex2D(_MainTex , coords.xy - _AberrationOffset);
//Green Channel
float4 green = tex2D(_MainTex, coords.xy );
//Blue Channel
float4 blue = tex2D(_MainTex, coords.xy + _AberrationOffset);
float4 finalColor = float4(red.r, green.g, blue.b, 1.0f);
return finalColor;
}
ENDCG
}
}
}
Code: Select all
hotlineShader = love.graphics.newShader[[
extern Image _MainTex;
number _AberrationOffset;
vec4 frag(v2f_img i) : COLOR
{
vec2 coords = i.uv.xy;
_AberrationOffset /= 300.0f;
//Red Channel
vec4 red = Texel(_MainTex , coords.xy - _AberrationOffset);
//Green Channel
vec4 green = Texel(_MainTex, coords.xy );
//Blue Channel
vec4 blue = Texel(_MainTex, coords.xy + _AberrationOffset);
vec4 finalColor = vec4(red.r, green.g, blue.b, 1.0f);
return finalColor;
}
vec4 effect( vec4 color, Image texture, vec2 texture_coords, vec2 screen_coords ){
}
]]
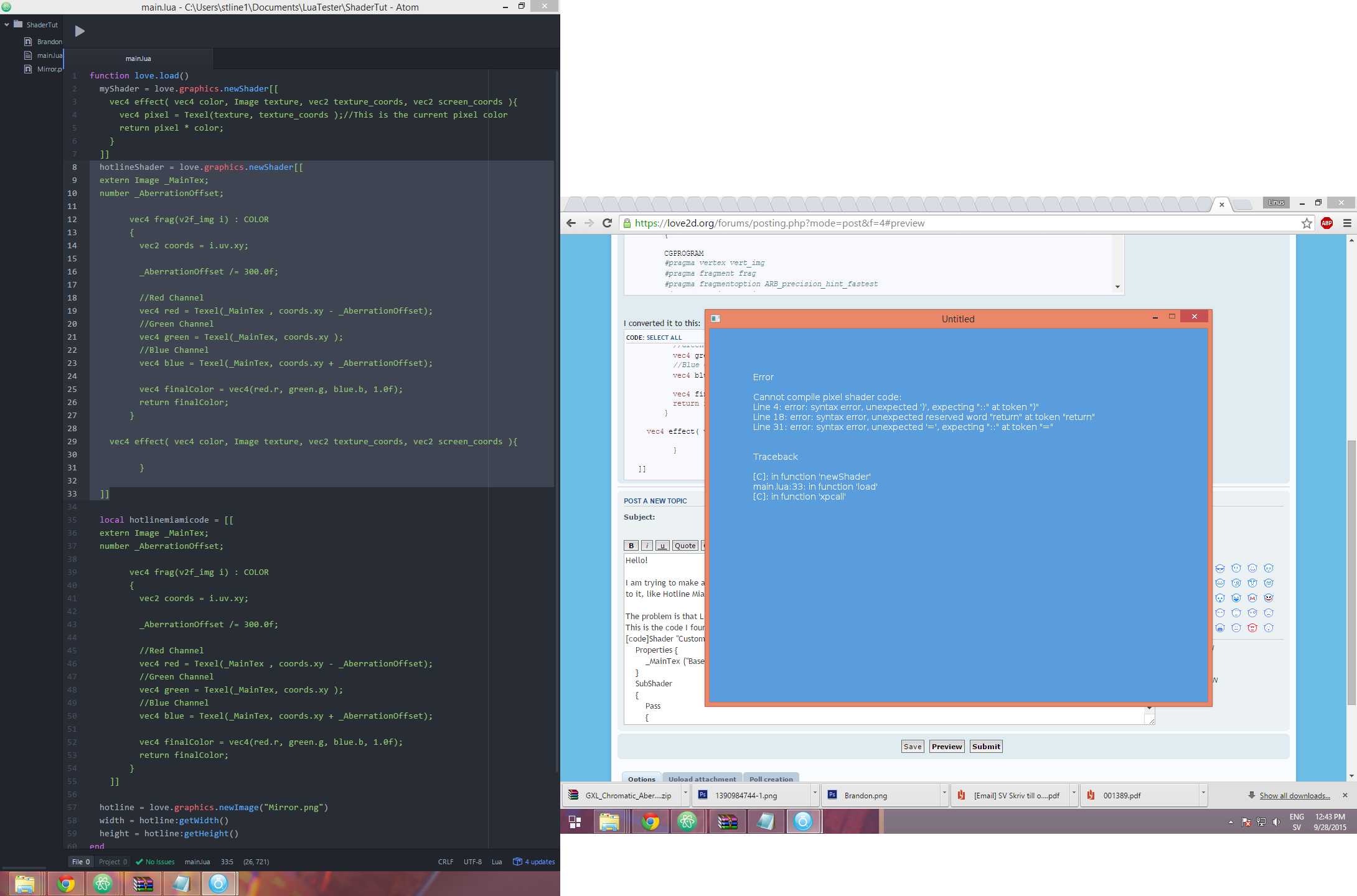
Any help is appreciated, I have only written a bloom shader before and that was in HLSL and not in some "embedding kind of GLSL" style that Lua Löve2D has.
EDIT1:
I have made a chromaticAberration.glsl file which I load as shader and it does this:
Code: Select all
float _AberrationOffset;
vec4 effect(vec4 col, Image texture, vec2 texturePos, vec2 screenPos)
{
vec2 coords = texturePos;
_AberrationOffset /= 300.0f;
vec4 pixel = texture2D(texture, texturePos);
//Red Channel
vec4 red = texture2D(texture , coords - _AberrationOffset);
//Green Channel
vec4 green = texture2D(texture, coords );
//Blue Channel
vec4 blue = texture2D(texture, coords + _AberrationOffset);
vec4 finalColor = vec4(red.r, green.g, blue.b, 1.0f);
return finalColor;
}