i have a problem...
i created a game minecraft-like but when I click on the edge of the world it's an error: attempt to index a nil value
code:
Code: Select all
function love.load()
map = {}
inv = {}
size = 16
maxStack = 64
selected = 0
invLen = 8
ver = "1.0"
id = ""
mx = 0
my = 0
w = math.floor(768 / size)
h = math.floor(576 / size)
love.window.setMode(768, 576)
love.window.setTitle("Cube2D "..ver)
isblock = true
image = 0
stack = 1
--blocks
air = {}
air[image] = love.graphics.newImage("assets/blocks/air.png")
air[stack] = 0
air[id] = "air"
air[isblock] = false
grass = {}
grass[image] = love.graphics.newImage("assets/blocks/grass.png")
grass[stack] = 0
grass[id] = "grass"
grass[isblock] = true
dirt = {}
dirt[image] = love.graphics.newImage("assets/blocks/dirt.png")
dirt[stack] = 0
dirt[id] = "dirt"
dirt[isblock] = true
stone = {}
stone[image] = love.graphics.newImage("assets/blocks/stone.png")
stone[stack] = 0
stone[id] = "stone"
stone[isblock] = true
bedrock = {}
bedrock[image] = love.graphics.newImage("assets/blocks/bedrock.png")
bedrock[stack] = 0
bedrock[id] = "bedrock"
bedrock[isblock] = true
cactus = {}
cactus[image] = love.graphics.newImage("assets/blocks/cactus.png")
cactus[stack] = 0
cactus[id] = "cactus"
cactus[isblock] = true
trunck = {}
trunck[image] = love.graphics.newImage("assets/blocks/trunck.png")
trunck[stack] = 0
trunck[id] = "trunck"
trunck[isblock] = true
leaves = {}
leaves[image] = love.graphics.newImage("assets/blocks/leaves.png")
leaves[stack] = 0
leaves[id] = "leaves"
leaves[isblock] = true
sapling = {}
sapling[image] = love.graphics.newImage("assets/blocks/sapling.png")
sapling[stack] = 0
sapling[id] = "sapling"
sapling[isblock] = false
--items
pickaxe = {}
pickaxe[image] = love.graphics.newImage("assets/items/pickaxe.png")
pickaxe[stack] = 0
pickaxe[id] = "pickaxe"
pickaxe[isblock] = false
generateLevel()
newInv()
inv[0] = stone
inv[1] = sapling
inv[0][stack] = 2
inv[1][stack] = 2
mod_init()
end
function addItem(item)
for i=0, invLen do
if inv[i][image] == item[image] then
inv[i][stack] = inv[i][stack] + 1
return
end
end
for i = 0, invLen do
if inv[i][image] == air[image] then
inv[i] = item
inv[i][stack] = inv[i][stack] + 1
return
end
end
end
function newInv()
for i = 0, invLen do
inv[i] = air
end
end
function generateLevel()
for x = 0, w do
map[x] = {}
for y = 0, h do
map[x][y] = air
end
end
for x = 0, w do
for y = 0, h do
end
end
for x = 0, w do
for y = 0, h do
if map[x][y] ~= bedrock then
if y > 16 then
map[x][y] = dirt
end
if map[x][y] == dirt and map[x][y-1] == air then
map[x][y-math.random(0, 1)] = grass
end
if map[x][y] == grass and map[x][y-1] ~= air then
map[x][y] = dirt
end
end
end
end
for x = 0, w do
for y = 0, h do
if map[x][y] == grass then
map[x][y+4] = stone
end
if map[x][y] == stone then
if map[x][y] ~= bedrock then
map[x][y+1] = stone
end
end
end
end
for x = 0, w do
for y = 0, h do
if math.random(0, 20) == 5 and map[x][y] == grass then
map[x][y] = sapling
end
end
end
end
function love.update(dt)
mx, my = love.mouse.getPosition()
mx = (math.ceil(mx / size) - 1)
my = (math.ceil(my / size) - 1)
if love.mouse.isDown("l") and map[mx][my] ~= air then
if map[mx][my] == leaves then
addItem(sapling)
end
addItem(map[mx][my])
map[mx][my] = air
end
if love.mouse.isDown("r") and isblock then
if map[mx][my] == air then
map[mx][my] = inv[selected]
map[mx][my][stack] = map[mx][my][stack] - 1
end
end
mod_update()
end
function love.draw()
mx, my = love.mouse.getPosition()
mx = (math.ceil(mx / size) - 1)
my = (math.ceil(my / size) - 1)
love.graphics.setBackgroundColor(0, 50, 255)
for x = 0, w do
for y = 0, h do
love.graphics.draw(map[x][y][image], x * size, y * size)
if map[x][y] == dirt and map[x][y-1] == air then
if math.random(0, 20) == 10 then
map[x][y] = grass
end
end
if map[x][y] == grass and map[x][y-1] ~= air and map[x][y-1] ~= flowers then
map[x][y] = dirt
end
end
end
love.graphics.setColor(255,255,255,60)
love.graphics.rectangle('fill', mx * size, my * size, size, size)
love.graphics.setColor(255,255,255)
for i=0, invLen do
if inv[i][stack] <= 0 then
inv[i] = air
end
love.graphics.setColor(0,50,255)
love.graphics.rectangle("line", (selected*34)+37, 549, 19, 20)
love.graphics.setColor(0,50,100)
love.graphics.rectangle("fill", (i*34)+38, 550, 18, 18)
love.graphics.setColor(255, 255, 255)
love.graphics.draw(inv[i][image], (i*34)+39, 551)
love.graphics.setColor(255, 255, 255)
if inv[i][stack] > 1 then
love.graphics.setColor(0, 0, 0)
love.graphics.print(inv[i][stack], (i*34)+38, 536)
love.graphics.setColor(255, 255, 255)
end
end
for x = 0, w do
for y = 0, h do
if map[x][y] == sapling and math.random(0, 100) == 5 then
map[x][y] = trunck
map[x][y-1] = trunck
map[x][y-2] = trunck
map[x][y-3] = trunck
map[x][y-4] = trunck
map[x][y-5] = trunck
map[x][y-6] = trunck
map[x-1][y-4] = leaves
map[x+1][y-4] = leaves
map[x-1][y-5] = leaves
map[x+1][y-5] = leaves
map[x-1][y-6] = leaves
map[x+1][y-6] = leaves
map[x][y-7] = leaves
map[x+2][y-5] = leaves
map[x-2][y-5] = leaves
end
end
end
mod_draw()
end
function love.keypressed(key)
if key == "1" then
selected = 0
elseif key == "2" then
selected = 1
elseif key == "3" then
selected = 2
elseif key == "4" then
selected = 3
elseif key == "5" then
selected = 4
elseif key == "6" then
selected = 5
elseif key == "7" then
selected = 6
elseif key == "8" then
selected = 7
elseif key == "9" then
selected = 8
end
end
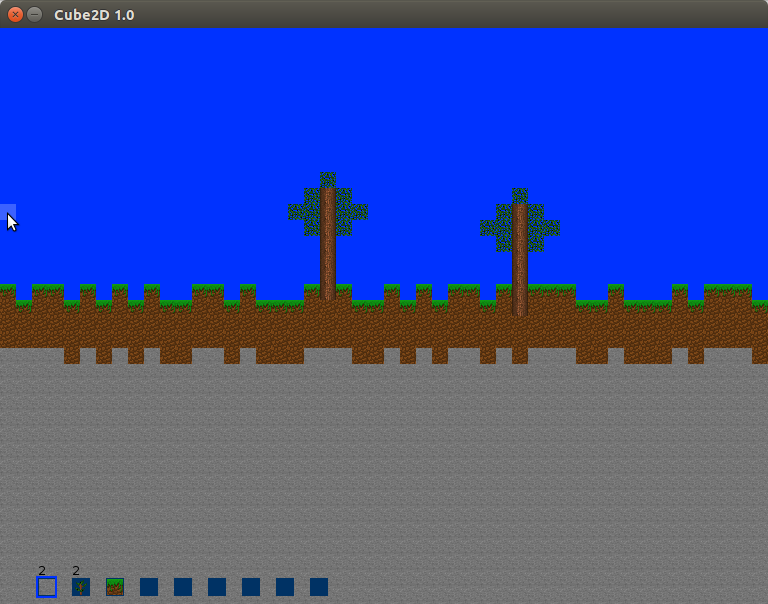
error:
Code: Select all
Error: main.lua:178: attempt to index a nil value
stack traceback:
main.lua:178: in function 'update'
[string "boot.lua"]:442: in function <[string "boot.lua"]:413>
[C]: in function 'xpcall'