I made a tiny library that lets you easily put basic sliders in your game. It gives you customizable sliders that can manipulate properties, like audio volume.
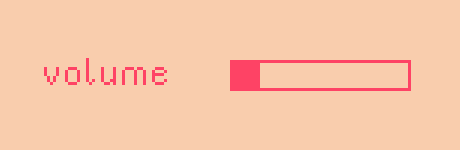
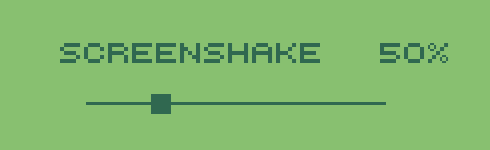
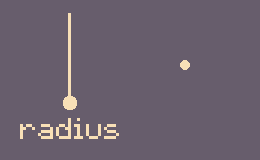
Example
Code: Select all
require 'simple-slider'
function love.load()
music = love.audio.newSource('music.ogg')
music:play()
-- create a new slider
volumeSlider = newSlider(400, 300, 300, 0.5, 0, 1, function (v) love.audio.setVolume(v) end)
end
function love.update(dt)
-- update slider, must be called every frame
volumeSlider:update()
end
function love.draw()
love.graphics.setBackgroundColor(249, 205, 173)
love.graphics.setLineWidth(4)
love.graphics.setColor(254, 67, 101)
-- draw slider, set color and line style before calling
volumeSlider:draw()
end
Download simple-slider.lua and place it in your game directory. Make sure to require it.
Code: Select all
require 'simple-slider'
Code: Select all
slider = newSlider(x, y, length, value, min, max, setter, style)
-- x: x position of center of slider
-- y: y position of center of slider
-- length: length of slider track
-- value: initial value for property
-- min: minimum value for property
-- max: maximum value for property
-- setter: function called when slider is updated, supplied with current slider value
-- style: optional table of style flags
-- width: width of knob, defaults to 0.1 of slider length
-- orientation: 'horizontal' or 'vertical', defaults to 'horizontal'
-- track: track style, 'rectangle' or 'roundrect' or 'line', defaults to 'rectangle'
-- knob: knob style, 'rectangle' or 'circle', defaults to 'rectangle'
Code: Select all
slider:update()
-- or optionally
slider:update(mouseX, mouseY, mouseDown)
-- (this allows you to supply your own parameters for mouse position and state - useful if your draw calls are being offset, or you want to use a controller for input)
Code: Select all
slider:draw()
Code: Select all
slider:getValue()
Download