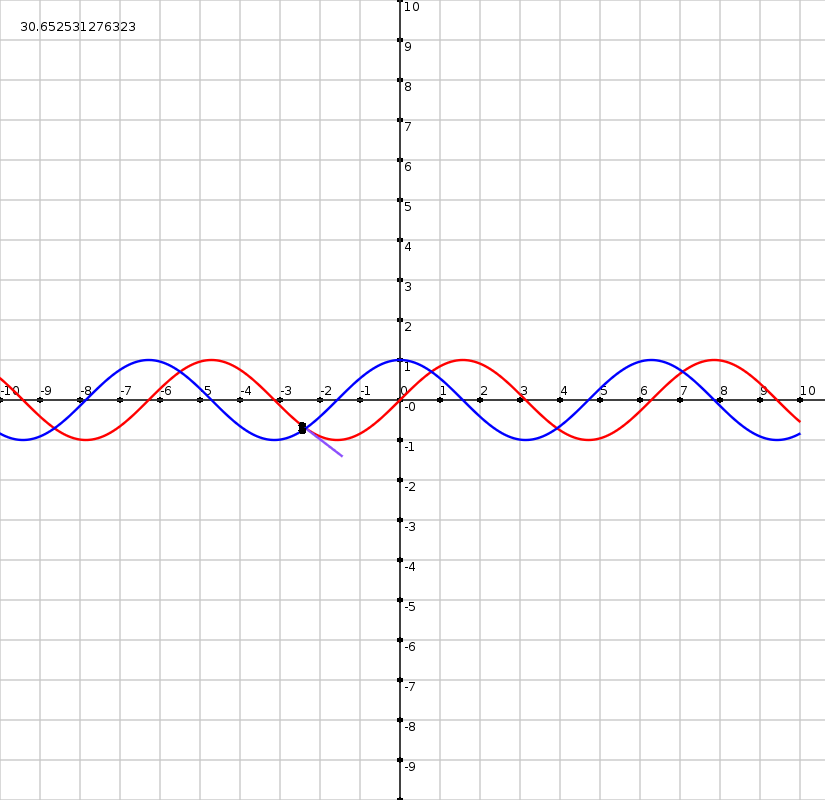
Right mouse button to move the ball, left mouse button to pause.
You can add graphs by adding
Code: Select all
f[number](x) return --[[do stuff with x here]] end
Code: Select all
f[number]_ (x) etc.
Code: Select all
function love.load()
love.window.setMode( 800, 800)
colors = {
{255,0,0},
{0,255,0},
{0,0,255},
{255,0,255},
{255,255,0},
{0,255,255}
}
width = 400
widthN = 10*(width/400)
mod = (width/widthN)
z = -6
speed = 4
love.graphics.setBackgroundColor(255, 255, 255)
end
function love.update(dt)
if not love.mouse.isDown("l") and not love.mouse.isDown("r") then
z = z + dt * speed
end
if love.mouse.isDown("r") then
z = (love.mouse.getX()-400)/mod
end
end
function love.draw()
love.graphics.translate(width,width)
love.graphics.setColor(0,0,0)
love.graphics.setLineWidth(1)
for i=-widthN,widthN do
love.graphics.setColor(200,200,200)
love.graphics.line(-width,i*mod,width+25,i*mod)
love.graphics.line(i*mod,-width,i*mod,width+25)
love.graphics.setColor(0,0,0)
love.graphics.print(i,i*mod,-16)
love.graphics.circle("fill",i*mod,0,3,10)
love.graphics.print(-i,4,i*mod)
love.graphics.circle("fill",0,i*mod,3,10)
end
love.graphics.line(-width,0,width+25,0)
love.graphics.line(0,-width,0,width+25)
love.graphics.setLineWidth(2)
local F = 1
while(true) do
local f = _G["f" .. F]
local f_ = _G["f" .. F .. "_"]
if not f then break end
--RED
local col = 1 + ((F-1) % #colors)
love.graphics.setColor(unpack(colors[col]))
local line = {};
for i=-widthN*20,widthN*20 do
local x = i/20
local y = _G["f" .. F](x);
table.insert(line,x*mod)
table.insert(line,-y*mod)
end
love.graphics.line(line)
if f_ then
local x = z*mod
local y = -f(z)*mod
local dirY = -f_(z)*mod
love.graphics.setColor(0,0,0)
love.graphics.line(x,y,x+mod,y+dirY)
love.graphics.setColor(0,0,0);
love.graphics.circle("fill",x,y,4,10)
love.graphics.setColor(0,0,255)
local line = {};
for i=-widthN*20,widthN*20 do
local x = i/20
local y = f_(x)
table.insert(line,x*mod)
table.insert(line,-y*mod)
end
love.graphics.line(line)
x = z*mod
y = -f_(z)*mod
love.graphics.setColor(0,0,0)
love.graphics.circle("fill",x,y,4,10)
end
F = F + 1
end
end
function f1(x)
return math.sin(x)
end
function f1_(x)
return math.cos(x)
end
-- function f2(x)
-- return x^2
-- end
-- function f2_(x)
-- return 2*x
-- end
-- function f3(x)
-- return math.sqrt(x)
-- end
-- function f3_(x)
-- return 1/(2*math.sqrt(x))
-- end
function love.mousepressed(x,y,key)
if key == "wd" then
speed = math.max(0,speed - 0.10)
elseif key == "wu" then
speed = math.min(10,speed + 0.10)
end
end