I have found an issue and I can't seem to figure out how to fix it.
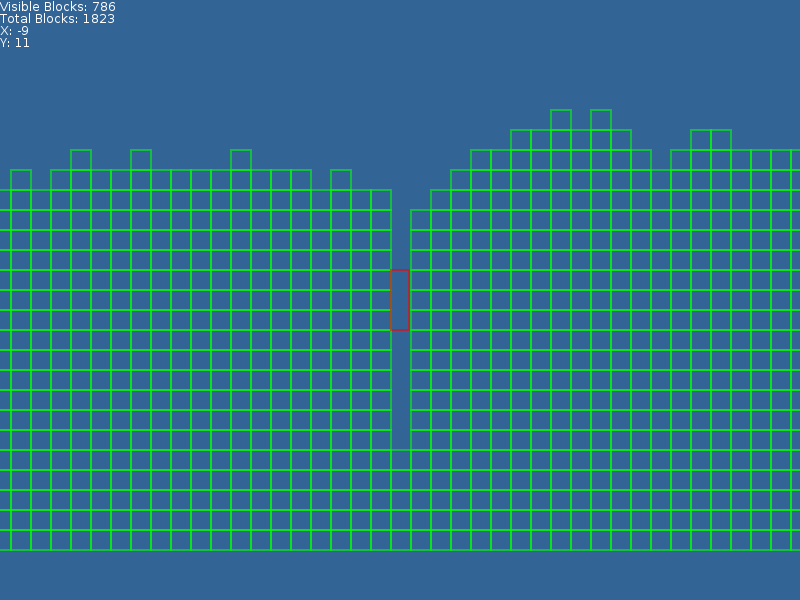
As you can see, when the player falls it gets stuck on the side of the blocks. I have further debugged this and it seems that, somehow, it is registering that it is touching the top of a block to form bottom collision for the player. I don't understand how this is possible. The player's collision box is eighteen pixels wide while the space between two blocks is twenty pixels wide so I don't believe it could be an issue of the player getting stuck. Here is the code which checks collision as well as the code that applies it.
The code which checks collisions between two objects:
Code: Select all
function checkCollision(object1, object2)
local right = (object1.x + object1.w) - object2.x
local left = (object2.x + object2.w) - object1.x
local bottom = (object1.y + object1.h) - object2.y
local top = (object2.y + object2.h) - object1.y
if right < left and right < top and right < bottom then
return "right"
elseif left < top and left < bottom then
return "left"
elseif top < bottom then
return "top"
else
return "bottom"
end
end
Code: Select all
for k, v in ipairs(objects) do
if player.x + player.w >= v.x and player.x <= v.x + v.w and player.y + player.h >= v.y and player.y <= v.y + v.h then
local collision = checkCollision(player, v)
if collision == "bottom" then
player.y = v.y - player.h
player.collisions.bottom = true
end
if collision == "right" then
player.x = v.x - player.w
end
if collision == "left" then
player.x = v.x + v.w
end
if collision == "top" then
player.y = v.y + v.h
end
end
end