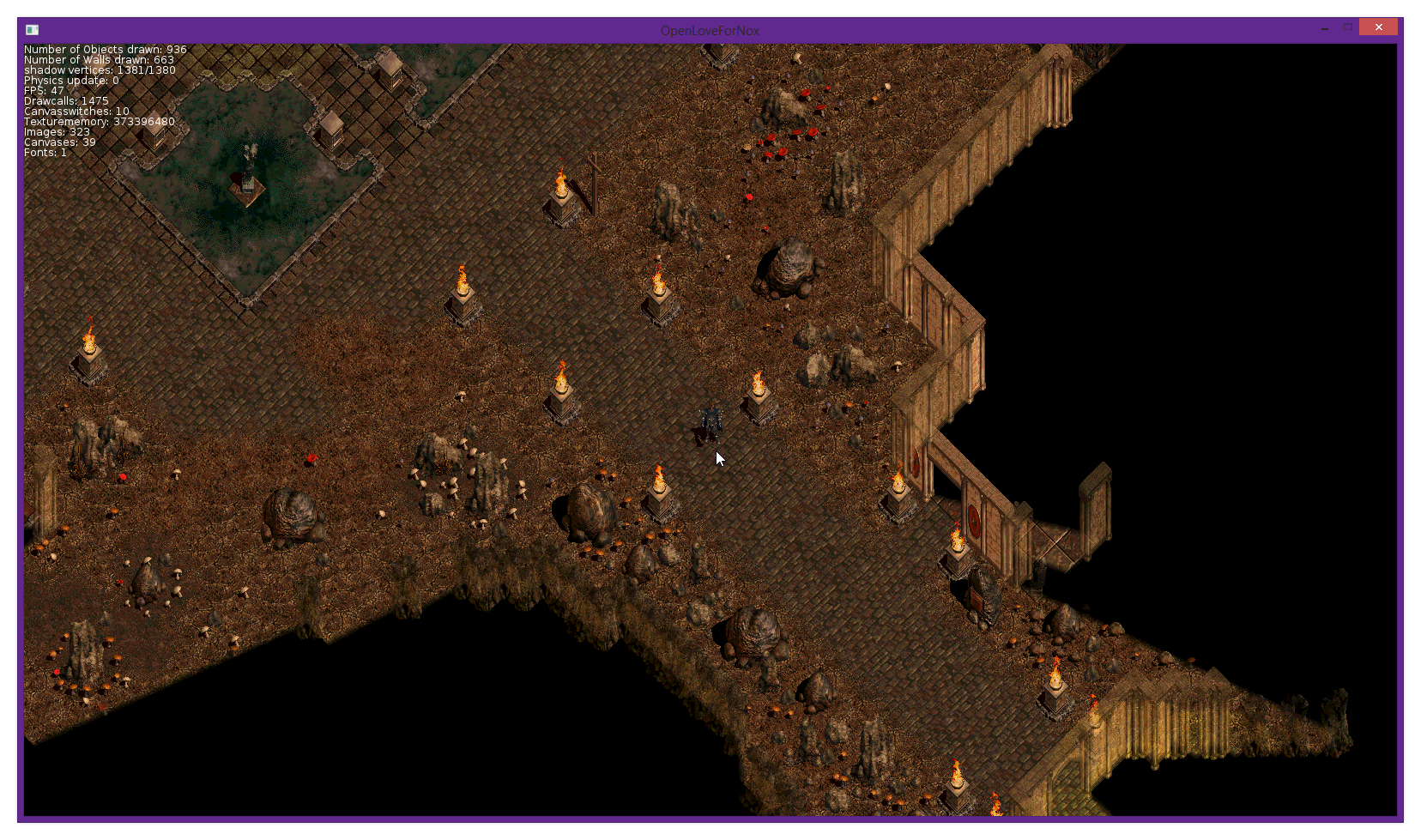
I am experiencing a performance issues when dealing with a "Large" amount of objects ... Or in this case, somewhere around a thousand.
The "Draw Objects" routine, that draws all non static objects, within the current position of the spatialhash takes up around 50% of the frametime, and looks something like this:
Code: Select all
for i=1, #objects do
local obj = objects[i]
if(obj.renderer) then
obj.renderer:draw(obj)
elseif(obj.draw) then
obj:draw()
end
end
local gdraw = love.graphics.draw
function StaticDraw:draw(obj) -- An example of an object render
self.shader:send("type46",false)
self.shader:send("pos",{camera:worldToLocal(obj.x,obj.y)})
gdraw(obj.img, obj.quad, round(obj.x + obj.drawOffsetX), round(obj.y + obj.drawOffsetY))
end
Even when the Nvida GPU and the CPU is significantly worse then the AMD counterpart.
When inspecting the project using CodeXL (aka gDebugger) i find that the 1500 in engine drawcalls are unrolled into
16 thousand OpenGL calls. With a LOT of seemingly redudant state changes such as:
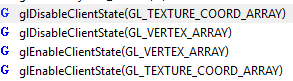
At 1500 drawcalls, totaling at 16k opengl calls. I would be expecting performance to be somewhat higher then this. And i am really wondering if i am missing something here or ran into a bug of some kind, or if this is actually expected
Are there any ways to improve the performance without going into spritebatches, or coming up with a native solution?